A guide to organization modeling
This guide surfaces complexities and implementation details for supporting organization modeling as part of the authentication and authorization layer for apps.
Users and teams and orgs, oh my! How to do organization modeling
Organizational modeling - or how your app handles users, teams, and the relationships between the two - is an important but oft overlooked part of building authentication and authorization into your app. Simply put, if you ever want to sell to an enterprise your backend needs to be ready to support complex models of users and teams.
Organizational modeling is actually pretty simple in 90% of cases, but when you add different types of relationships and SSO into the mix, things get complicated fast. This post will walk through how to think about organizational modeling for your app and a few practical considerations for implementation.
But first, a common disclaimer: every organization and business are different. We've tried to make as few assumptions as possible. Should any of our assumptions not align with the specifics of your business, we hope that the underlying concepts will still prove to be valuable.
How organization modeling works conceptually
Organization modeling is sort of like an ogre, which is like an onion, in that it has layers. The basic concepts are simple, but as you go deeper, edge cases (especially SSO) can make things highly complex. Let's start with two basic ideas: the user and the organization.
A user maps to someone with a login. A person can usually have multiple user accounts, since they can have multiple logins or identities. But as far as your app is concerned, a user maps 1:1 with a login.
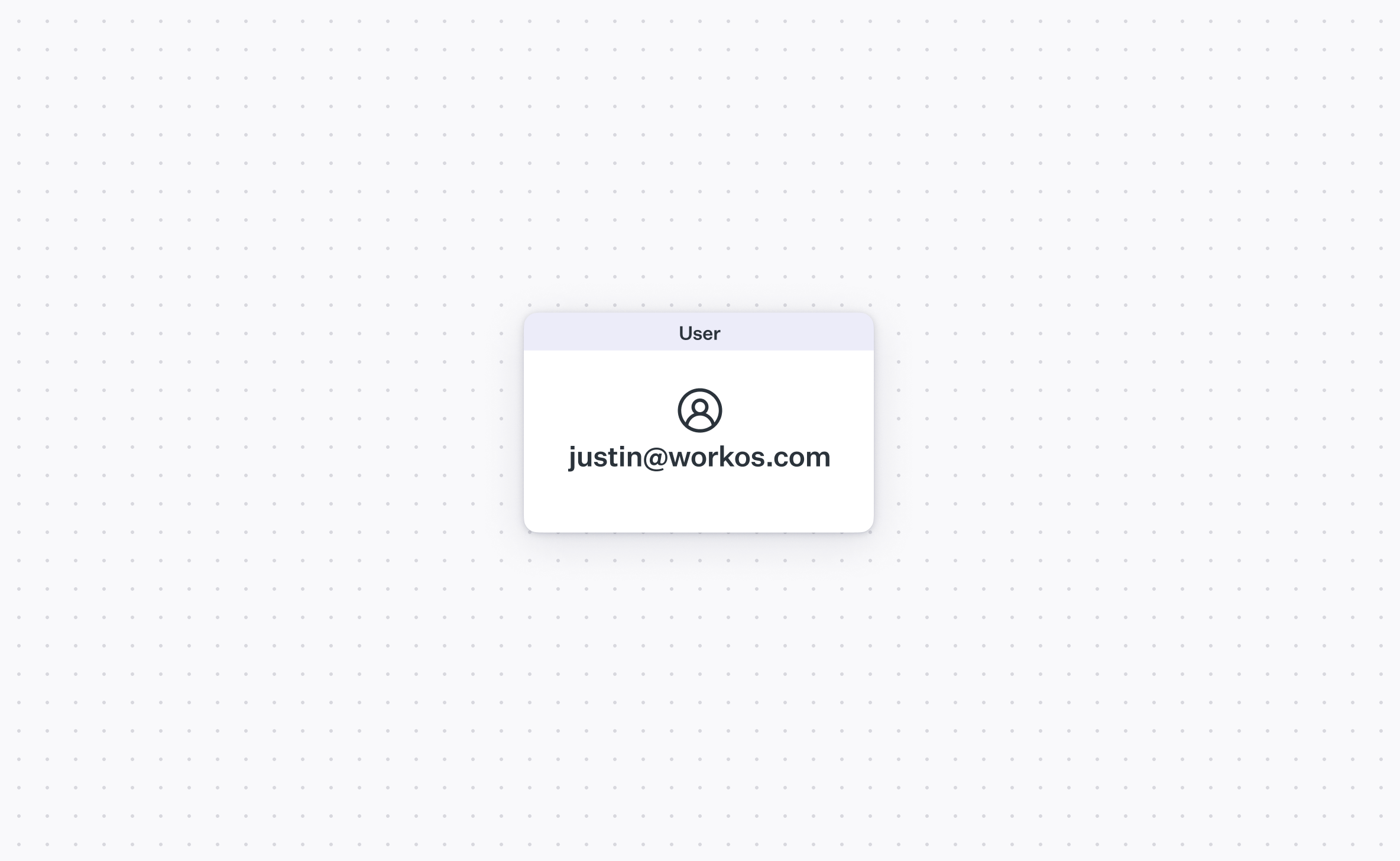
An organization is a group of 1 or more users who shares access to the same resources, or artifacts. If a user maps to someone with a login, you can sort of think about an organization mapping to an IT admin.
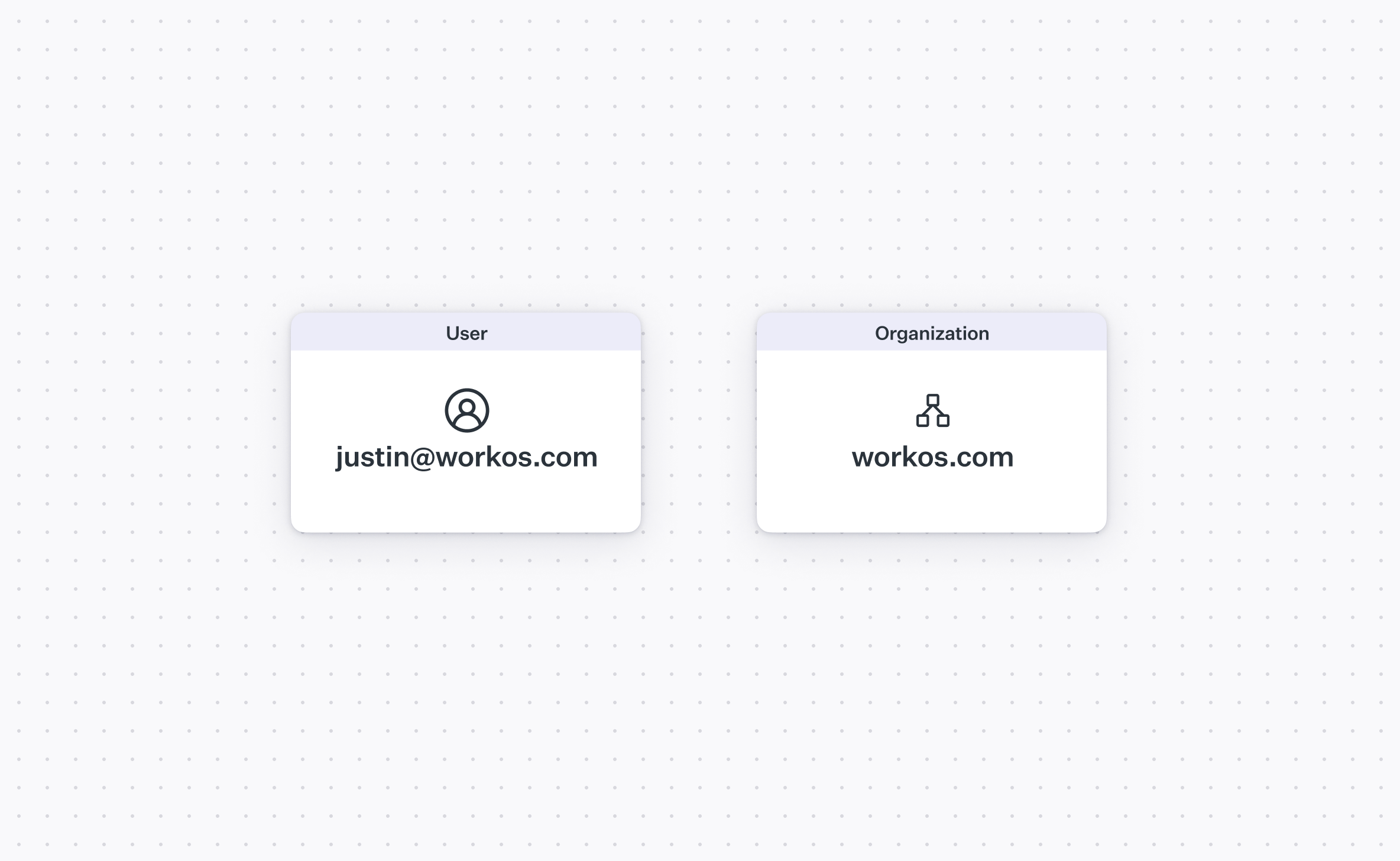
An artifact can be anything really, it depends on your app. At Digital Ocean (author used to work here), artifact was a cloud resource like a VM, managed database, firewall, etc. At Figma, an artifact might be a shared marketing folder or blog assets. Note that these artifacts can "belong" to a particular user in an organization and be inaccessible to other users in that organization (like a private file).
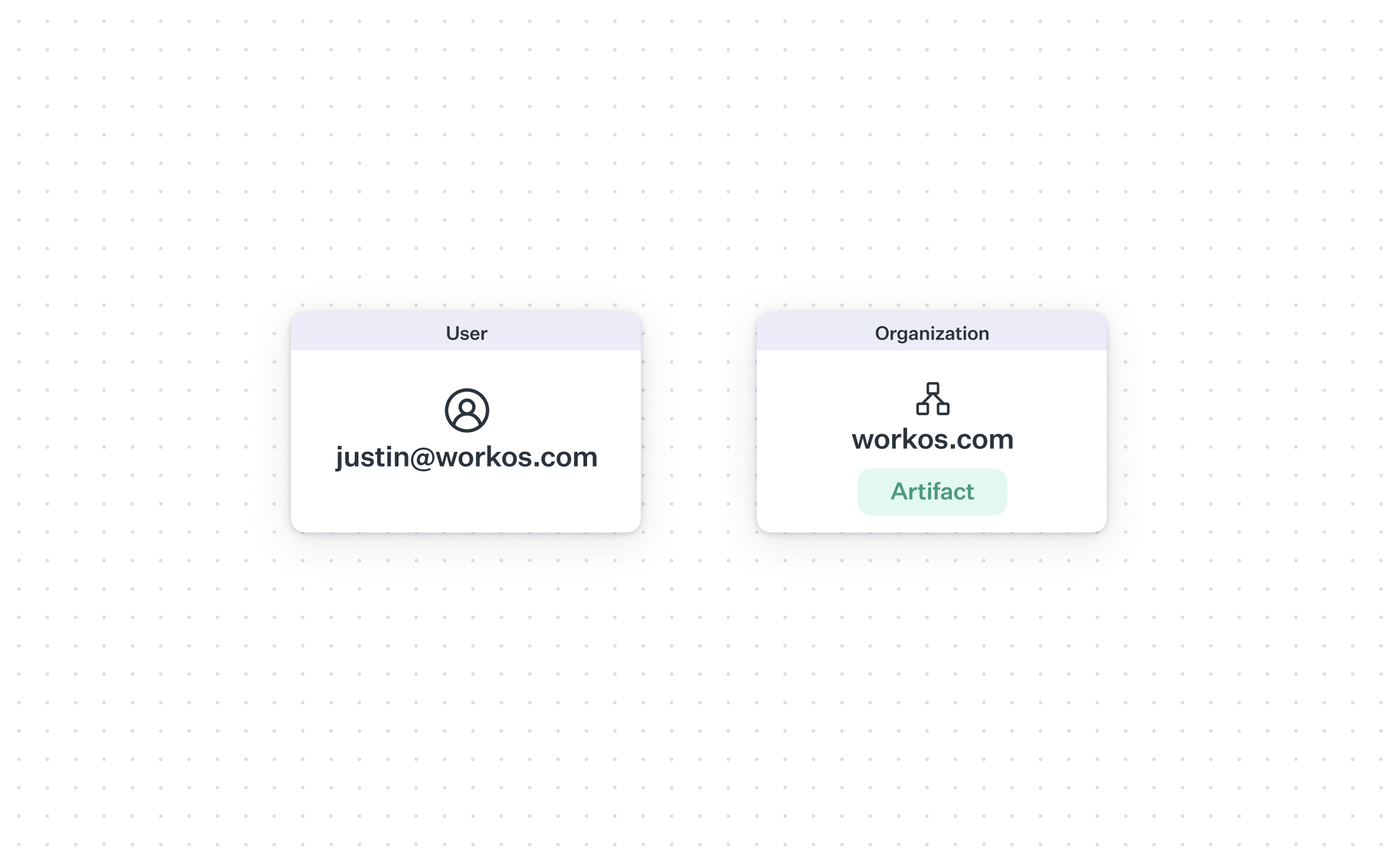
In some implementations, an organization will have a unique domain, e.g. a multi-tenant SaaS application where each organization gets a subdomain or a custom domain for the entire deployment.
So, can a user be a part of multiple organizations? The relationship between a user and organization can be modeled in two major ways. In a many-to-one relationship, an organization can have many users but a user can only be a member of one organization.

You can also model this relationship as many-to-many, where a user can be part of multiple organizations. The main user case for this kind of relationship is contractors and agencies, who have multiple clients and need access to all of their workspaces from a single user account.
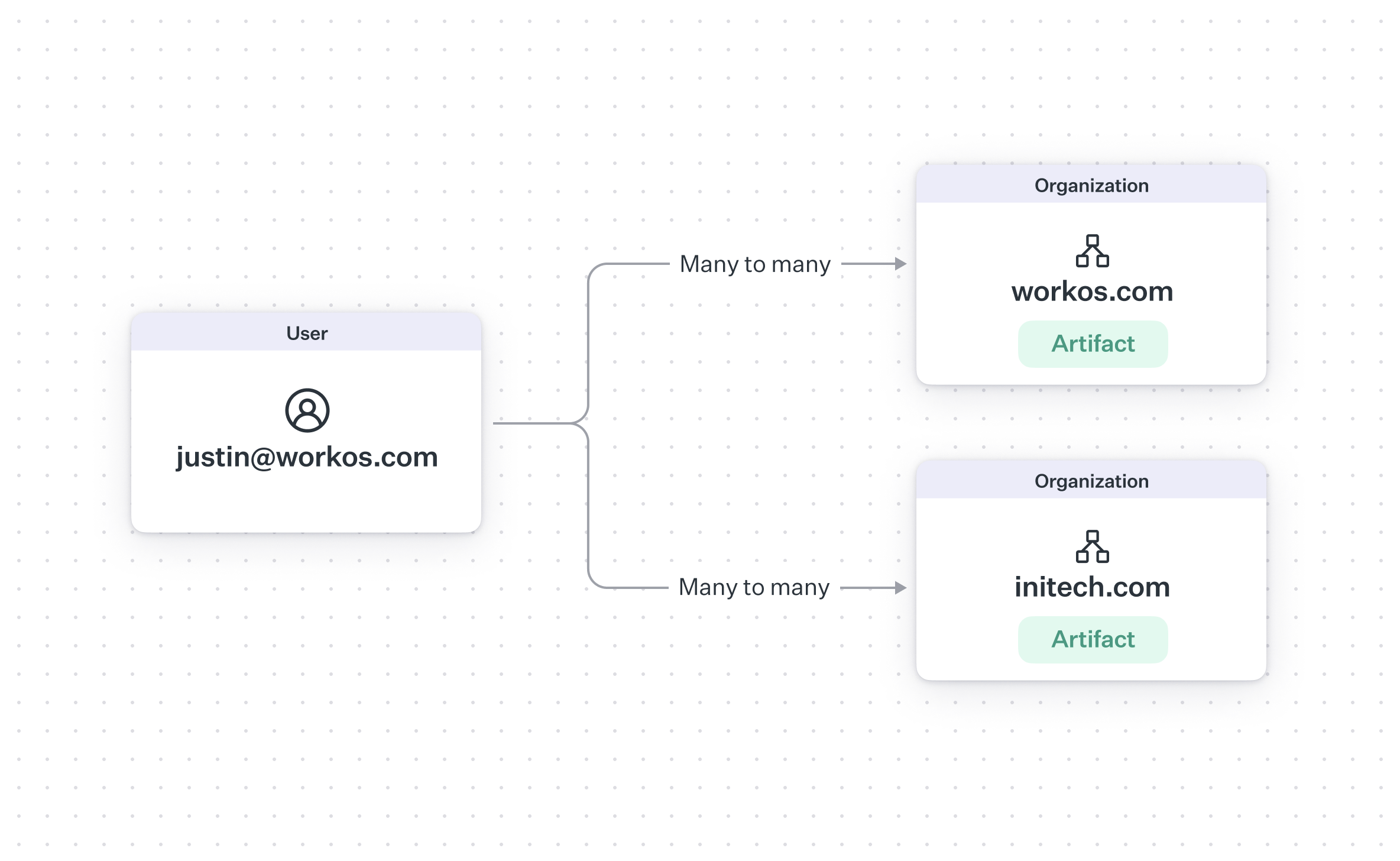
There's a third, lower level concept that you can think of as a workspace: a sublevel to an organization that organizes the users and artifacts inside it.
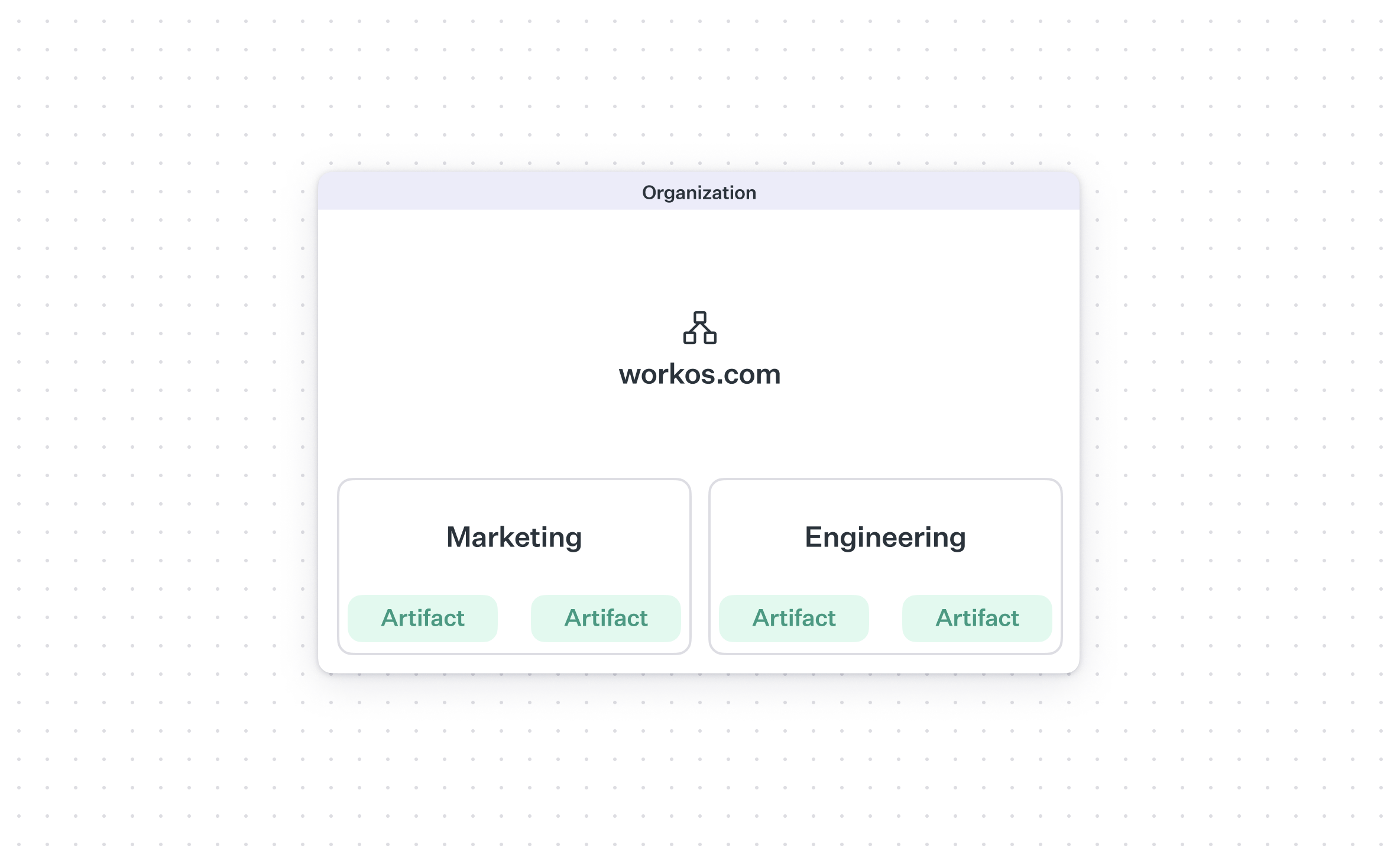
The easiest way to think about this is multiple teams at one customer. It's safe to assume that all 200K employees at Microsoft aren't using the same dumping ground for Figma files. There's probably a separate workspace for Marketing vs. Sales vs. Engineering, and even within those, several more subdivisions.
Slack is a good example of the confluence of all of these ideas. You can have multiple Slack accounts across different email addresses: each of those would represent a user. Each of those users can be a part of multiple workspaces. Slack actually uses the "workspace" word explicitly, but it more closely maps to the "organization" concept as defined in this blog post.
Workspaces are a lower level concept than a user or an org, because they don't have any authentication implications (purely authorization). Both users and orgs, as well as how they relate to each other, will have major impact on how you built you auth schemes, especially once we get into the wacky, wild world of SSO and SAML.
How organization modeling works, practically
With that conceptual backing in mind, let's focus more on how you'd actually implement this on the backend (and to a lesser extent, the frontend).
Tables for users and organizations
Complexity level 0: you model users to organizations as many-to-one, and each user can only be in one organization. In that case, you just need two tables in your data model, one for users and one for organizations. Each row in the users table has a foreign key, let's call it organization_id, to a row in the organizations table.
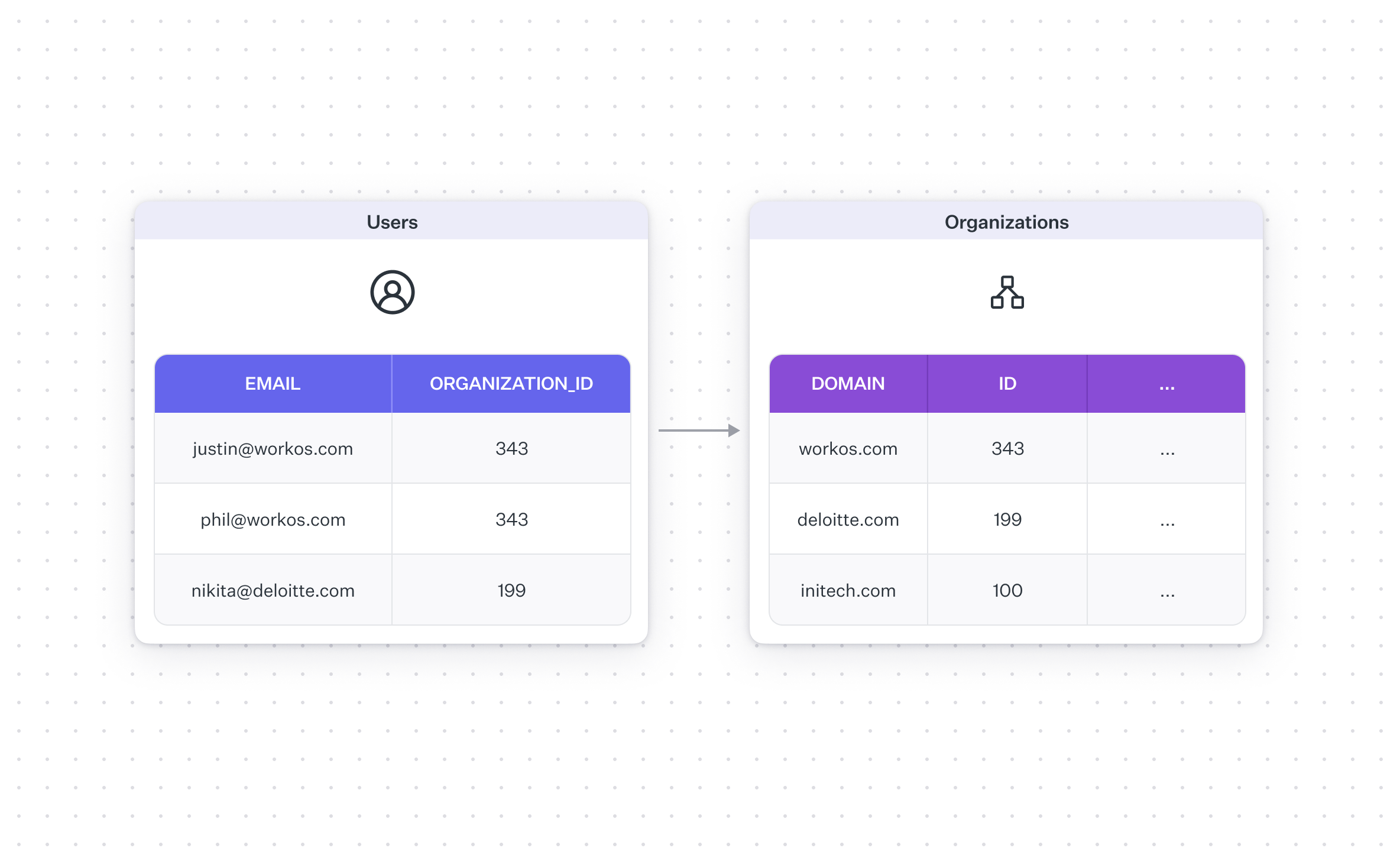
This model is simple and easy, especially for your analytics team that only needs to do one join.
A membership table for many-to-many
If you do decide to model users to organizations as many-to-many, you'll need a third table to keep track of the "memberships" of different users to different organizations. It should be something like one row per user per membership, with foreign keys out to both the user and organization tables.

Note that even if a user can belong to multiple organizations, there will usually be a primary organization that "owns" the user account, e.g. whoever owns the domain. If Nikita is a contractor at Deloitte and uses her Deloitte email to authenticate, then the Deloitte organization owns her account, even though she has access to other organizations (her clients). This has important implications for cases in which IT admins mandate certain authentication schemes (see below).
Choosing your organization on login
If you choose to support a user belonging to multiple organizations, you will need to build some way for them to choose which organization they want access at a given point in time. The most straightforward way to do this is on login:
- User authenticates (in whichever way their owning organization requires)
- User chooses which organization they want to access
Building an organization "switching" functionality once a user is already authenticated sounds nice on paper but is actually really difficult because...
Where it gets complicated: org switching and SSO
As with pretty much everything, when you invite SSO to the party you start to have trouble.
Organizational modeling use cases follow somewhat of bimodal distribution between single organization users and multi-organization ones. Most users will only belong to one organization, and they'll cause you and your overworked engineering team absolutely no further harm. Once they authenticate, you don't need to worry about them anymore. But a small group of dilettantes will demand access to more than one organization (reality is that this is a reasonable request). And it's that small group that causes you the most trouble, because they will create an SSO Snafu™.
What Snafu are we referring to? IT admins at larger organizations will usually require members of their organizations to authenticate in a specific way, namely SSO via their IdP like Okta. If a user is a member of multiple organizations, each with its own unique SSO scheme, how do you handle that as a developer? Consider the following example:
- Nikita is an employee of Deloitte. Her job is to consult and contract with other organizations.
- Her user account uses her Deloitte email, nikita@deloitte.com. Because the Deloitte organization owns the deloitte.com domain, it owns her user account.
- The IT admin for Deloitte requires all users to log in via SSO through their IdP, which is Amazon Cognito.
- Nikita is working on a project for Initech, so she has access to Initech's organization; still under her Deloitte email.
- The IT admin for Initech requires all users to log in via SSO through their IdP, which is Okta.
Because the Deloitte organization owns the deloitte.com domain, and thus Nikita's user account, she is required to log in via their SSO. But if she then wants to access artifacts from Initech, she's going to need to do more authentication and log in via their SSO. In other words, accessing resources from another organization requires her to essentially reauthenticate according to that organization's policies.
Situations like this, which are not entirely uncommon, add a high degree of complexity to organization mapping that isn't present in most of the ways that your users will take advantage of it.
They also make it very difficult to implement "switching" functionality. In Nikita's situation, the most straightforward way to handle things would be:
- Require her to "general purpose" authenticate as per the Deloitte IT admin's requirements (e.g. their SSO connection).
- If she wants to work on artifacts within the Deloitte organization, great, she's all set.
- If she wants to work on artifacts within the Initech organization, she's going to need to do more authentication.
So doing this flow on login, as opposed to after login, centralizes the logic and keeps the user experience consistent.
Now is as good of a time as any to mention that if you don't want to build all of this yourself, you can always use something open source like AuthKit.