Actions — customize AuthKit behavior in real-time
We often think of auth as a binary decision—allowed or denied—but what if you want to factor in private knowledge or custom logic at runtime? Actions let you change how WorkOS behaves and customize user registration and authentication logic with AuthKit. Actions are also free to all AuthKit customers.
What are Actions?
Actions let your application execute arbitrary logic to allow or deny a user's sign-up or authentication in real-time. You can use Actions to deny sign-ups from certain domains or users on a deny list. You can enforce custom authentication requirements, such as limiting the number of failed authentications to satisfy PCI-DSS. You can implement whatever custom logic you wish.
How they work
When an Action is enabled, AuthKit makes a request to your specified endpoint before completing a sign-up or authentication. AuthKit waits for your application to respond with a verdict: allow or deny.
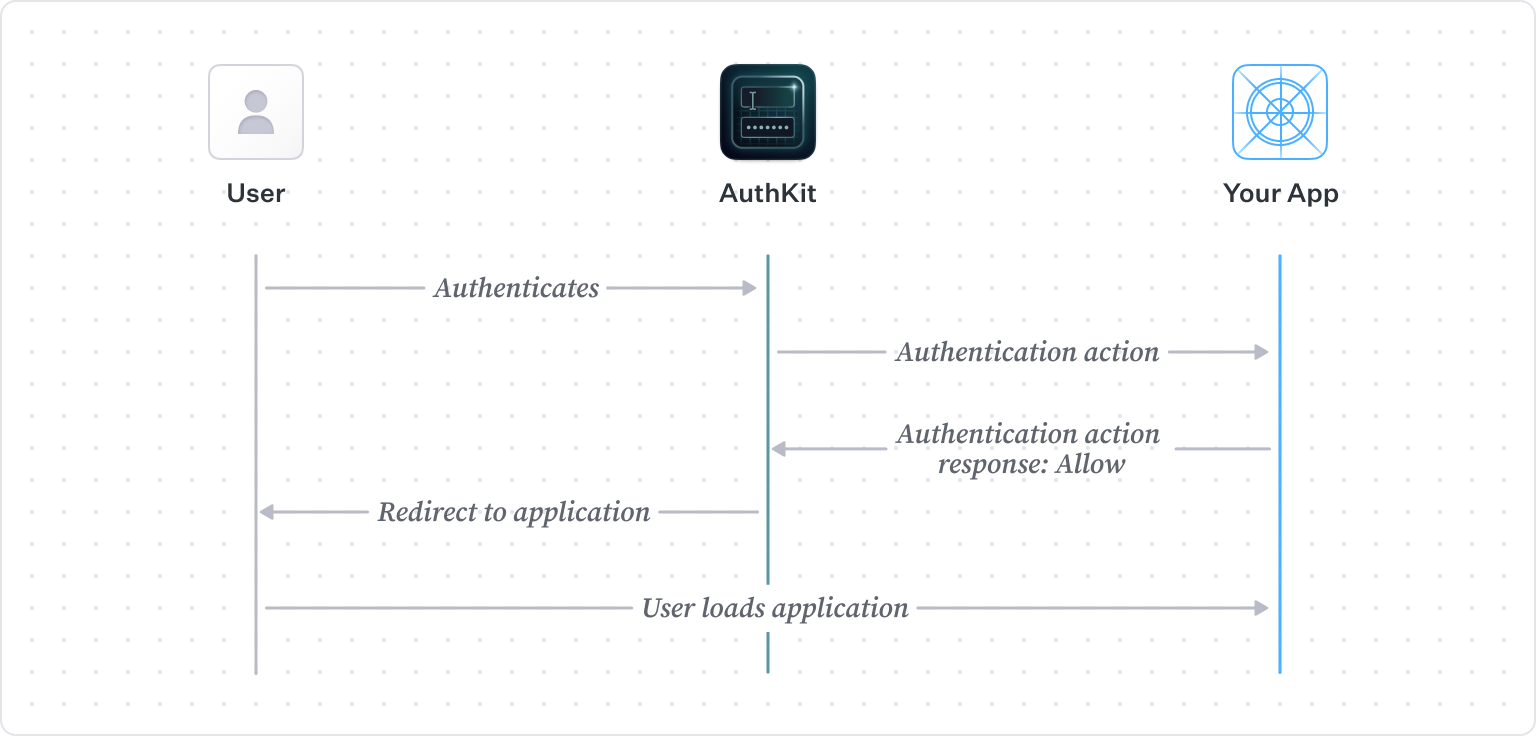
.webp)
To ensure Actions are secure, all Actions requests and responses include a cryptographic signatures. This means your application can verify that requests came from WorkOS. The WorkOS Node.js SDK comes with helper methods to simplify validation of the signature to ensure the action originated from WorkOS, and signing the response so WorkOS can verify it was your app that responded.
Using Actions
Configure an Action in the WorkOS dashboard by enabling the Action type, defining an endpoint for WorkOS to call, and selecting the error handling behavior.

Configurable error handling
No application has 100% uptime. If your application has a temporary outage, what should Actions do in this scenario? Your user might be authenticating into a highly secure environment where you would prefer to err on the side of caution and deny authentication.
In other cases, you may prefer to allow access and prioritize uptime for your application. When WorkOS can’t communicate with your application, every Action sets an error handling behavior to allow the flow to continue or deny by default. WorkOS also keeps an event log of all actions executed in the dashboard to assist in debugging unexpected behavior.
An example Action: block sign-ups from users with specific email domains
This example Action denies user registration from certain consumer email domains:
Actions or Events?
WorkOS also has an Events feature. Events communicate changes in WorkOS data to your application and are available through the Events API or Webhooks. So, when is it appropriate to use Events, and when should you use Actions?
Events communicate a change that’s already happened. They are a way of sharing information so your application can take action after the change. Common uses include: sending a welcome email after signup, updating user information in your CRM after a name change, or sending an email when a user signs in from a new device. Events don’t let you change core behaviors.
Actions should be used to change how WorkOS behaves. Rather than just receiving an update that a new user has signed up, your app can determine whether the user can sign up at all, for example, restricting sign-ups from certain regions.
What’s next
We are initially launching Actions for User Registration and Authentication. Actions are free for all AuthKit customers. We plan to add actions to let you customize more parts of the user lifecycle. If you’re interested in learning more, check out the actions documentation.
If you're interested in adding actions to other parts of the auth lifecycle, contact us.