Agno: The agent framework for Python teams
Agno is an open-source framework that helps you build clean, composable and Pythonic agentic applications with tools, memory and reasoning capabilities.
As AI agents move from experiments to real-world applications, teams are beginning to integrate them into internal tools, research workflows, and operations.
But building reliable, extensible agents still means stitching together wrappers, chains, and glue code. It’s messy, brittle, and hard to maintain.
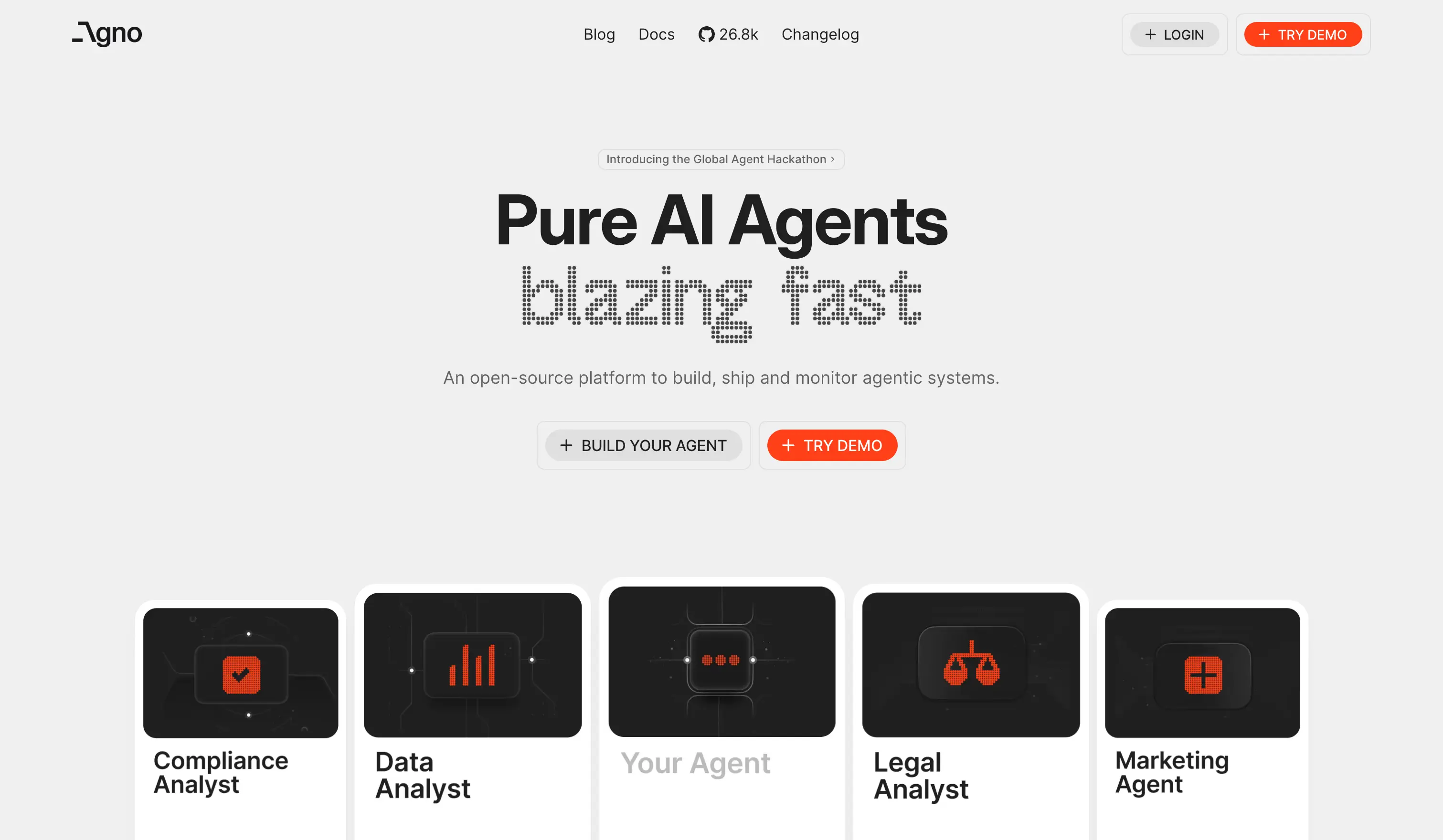
Agno is an open-source framework that brings structure to this process. It offers a clean, composable, and Pythonic approach to building AI agents with the tools, memory, and reasoning capabilities they need to support real work.
Key concepts
Declarative agent composition
Agno agents are constructed with a simple, declarative interface. You configure the model, memory, tools, and data sources with Python.Here’s what building an agent looks like:
agent = Agent(
model=OpenAI(id="gpt-01"),
memory=AgentMemory(),
storage=AgentStorage(),
knowledge=AgentKnowledge(
vector_db=PgVector(search_type="hybrid")
),
tools=[Websearch(), Reasoning(), Finance()],
description="You are the most advanced AI system."
)
Every component is a plug-and-play module, letting you customize behavior without vendor lock-in or framework ceremony.
Tools as first-class citizens
Tools are lightweight Python classes that expose specific capabilities to agents. They can be as simple as a function or as complex as a web-interacting bot.
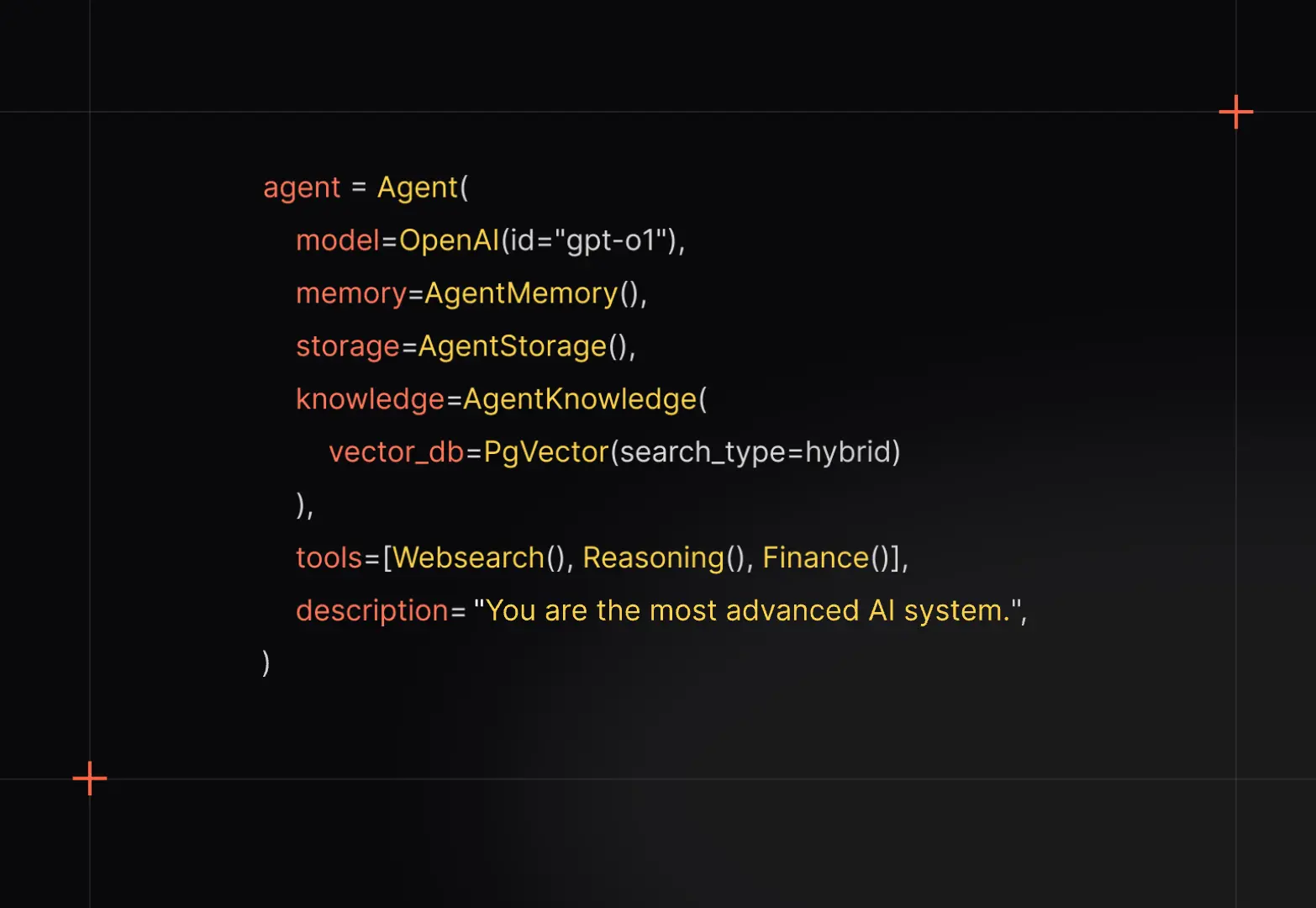
Built-in tools cover essentials like:
- Web search
- Financial data analysis
- Structured reasoning
- Code evaluation
- Custom workflows
You can mix and match tools or author your own with minimal boilerplate.
Knowledge and retrieval
Agno supports pluggable vector stores like PgVector and gives your agents access to hybrid search across structured documents, databases, and embeddings.
This enables more accurate answers, better grounding, and real information retrieval, not just in-context string stuffing.
Memory and storage
Short-term memory lets your agent track conversations and internal state across a session.
Long-term storage allows for a durable state, perfect for agents that evolve over time, run async tasks, or operate in scheduled workflows.
Transparent reasoning
Agno doesn’t hide how your agent thinks.
You can inspect reasoning traces, understand tool calls, and debug failures with minimal friction. This makes it perfect for production use cases where reliability and auditability matter.
Official examples
Here’s an example of an Agno agent that does research on a given stock. As you can see in the imports, Agno provides wrappers around foundational LLMs like Anthropic’s Claude or OpenAI models, as well as reasoning, finance and other tools:
from agno.agent import Agent
from agno.models.anthropic import Claude
from agno.tools.reasoning import ReasoningTools
from agno.tools.yfinance import YFinanceTools
agent = Agent(
model=Claude(id="claude-3-7-sonnet-latest"),
tools=[
ReasoningTools(add_instructions=True),
YFinanceTools(stock_price=True, analyst_recommendations=True, company_info=True, company_news=True),
],
instructions=[
"Use tables to display data",
"Only output the report, no other text",
],
markdown=True,
)
agent.print_response("Write a report on NVDA", stream=True, show_full_reasoning=True, stream_intermediate_steps=True)
Want to see Agno in action?
- ✍️ Blog post generator workflow
A multi-step agent that ideates, outlines, and drafts content using tools and memory. - 💰 Finance agent
A single-agent app with real-time financial data access, tool usage, and prompt-aware reasoning.
More examples at docs.agno.com/examples.
Who should use Agno?
Agno is ideal for:
- Python-native teams who want to build agentic systems without overhead
- Startups shipping internal copilots, analysts, or taskbots
- Engineers experimenting with RAG, workflows, or multi-agent setups
- Builders who want full control over how their agents think, act, and persist
It’s a strong fit for infra-minded teams that want a sane, composable foundation on which to build for months or years.
Getting started with Agno
- 🔗 Website & demo
- 💻 GitHub repo
- 🚀 pip install agno