Spot the bots: How to track malicious activity with JavaScript tagging
Tired of bots wreaking havoc on your website? Learn how JavaScript tagging can help you track suspicious behavior and stop malicious activity in its tracks.
The internet is a vast space where traffic can originate from legitimate users, automated bots, and malicious actors. Distinguishing between these sources is crucial for preventing fraud and enhancing overall website performance. There are several methods you can employ to detect and stop bots. One method you can use as part of your broader anti-bot strategy is JavaScript tagging. This way, you can use JavaScript to track user interactions and differentiate between human users and automated bots.
In this article, we’ll explore the role of JavaScript tagging in detecting bots, how it works, and how it can be implemented to track malicious activity on your website.
What is JavaScript tagging?
JavaScript tagging involves embedding small pieces of JavaScript code into web pages that allow site owners to track user interactions, gather data, and enhance the user experience. It can be used for various purposes such as website analytics, advertising tracking, session recording, and now, increasingly, for detecting malicious bot activity.
JavaScript tags are inserted into the HTML structure of web pages and are executed by the browser when the page loads.
For example, this simple script tracks when a user clicks anywhere on the webpage and logs it to the console:
Why bots are a threat
Before diving into how to detect bots with JavaScript tagging, let’s see why you should bother to do so.
Imagine you’ve just launched your new e-commerce website, and business is booming. Customers are browsing, adding products to their carts, and checking out. But one day, you notice something strange: sales are dipping, page load times are slowing down, and your website seems to be acting up. At first, you brush it off as a minor glitch, but as the days go by, the issues persist.
After some investigation, you discover that bots have invaded your site. They’re not just quietly browsing; they’re flooding your forms, attempting to break into accounts, and consuming your resources. These bots aren’t interested in buying anything—they’re just there to scrape your product data, overload your server, and even abuse your contact forms for spamming purposes. Your resources, which were meant to serve genuine customers, are now wasted on fake traffic.
Because of these bots, your site’s performance is taking a hit. Real users, frustrated by slow loading times, bounce off, and your conversion rates are dropping. Your reputation also begins to suffer; search engines notice the increased server load and reduce your ranking. Worse yet, because some bots are scraping your content and pricing, competitors could use that data to undercut your prices.
In the end, you realize that these bots are not just an inconvenience—they're a serious threat to your business. They’re costing you time, money, and the trust of your customers. Now, you understand why it's essential to keep them out: not only are they affecting your bottom line, but they also threaten the very integrity and success of your online presence.
Now that you understand the threat let's look at how JavaScript tagging can help in detecting these bots.
How JavaScript tagging detects malicious bots
JavaScript tagging can detect bots by tracking specific behaviors that are difficult for bots to replicate but natural for human users. These behaviors, while subtle, can act as red flags:
- Interaction speeds: One of the most common signs is the speed at which actions are performed. Human users tend to take their time—scrolling, clicking, typing—while bots, especially sophisticated ones, can perform these actions far too quickly. For example, filling out a form in a fraction of a second or submitting several actions at once is highly unusual for a person, but a bot can do it without breaking a sweat.
- Mouse movements: Another clear indicator is a lack of mouse movements. Bots, particularly simple ones, don’t have the ability to move a cursor around the page in a natural way. So, if a user visits a page and immediately interacts with it without any signs of scrolling or moving the mouse, it could be a bot.
- Overall behavior: Bots can exhibit erratic or repetitive actions, like repeatedly submitting forms or clicking the same buttons in quick succession, with no variation in the sequence. These kinds of repetitive behaviors are very different from how humans interact with a website, where actions typically vary and show signs of hesitation or deliberate choices. A user might move between pages, linger on certain elements, or exhibit an organic pattern of interaction. Bots, on the other hand, often follow a predictable and linear path. They’re highly efficient at what they do, but that efficiency is a giveaway. A user might take a while reading product descriptions before adding an item to their cart, while a bot might skip all of that and go straight for the checkout process.
Now, let’s see how we can use some of these red flags to identify bots with JavaScript tagging.
1. Tracking mouse movements
One way to spot a bot is by analyzing how a user interacts with your website. For example, a user’s mouse movements or scrolling behavior can tell you a lot about whether they’re human or automated. Bots, unlike humans, move in straight lines or skip sections of the page.
You could use a code snippet to track mouse movements on a page. If the mouse movements are too perfect (i.e., straight lines with no variation), it might be a bot.
Let’s see a simple example.
In this example, we are tracking the mouse movements and checking if the user is moving the mouse in a very predictable pattern. If the movements are too linear and lack any variation (which would be unusual for human users), it could indicate bot behavior.
2. Tracking scroll behavior
Humans usually scroll in a somewhat random pattern, often pausing to read content. Bots, however, tend to scroll at a consistent speed or skip large sections of a page. By tracking the scrolling behavior of users, we can identify potential bots.
Here’s a code snippet that tracks scroll behavior on the page.
In this example, we measure the time between scroll events to determine the scroll speed. If a user is scrolling too fast (for example, less than 50 milliseconds between scroll events), it may indicate automated behavior.
3. Form submission behavior
Bots tend to submit forms more quickly than humans and often in a consistent manner. This can be spotted by tracking the time it takes between form inputs and submission.
Here’s a code snippet that tracks form submission speed.
This code tracks the time taken to fill out and submit a form. If the form is submitted in less than a second, it's a strong indicator that a bot may be involved, since a human would typically take longer to fill out and submit a form.
4. Browser fingerprinting
Another method for detecting bots is browser fingerprinting. By gathering information such as the user agent, screen resolution, and installed plugins, you can create a unique fingerprint for each visitor. Bots tend to use the same fingerprint across multiple sessions, while human users usually exhibit more variability.
Here’s an example of basic browser fingerprinting.
This function generates a fingerprint based on the user’s browser details, screen resolution, and plugins. By storing and comparing fingerprints over time, you can detect if the same "user" is returning under different conditions, which may be indicative of a bot using rotation or automation.
Challenges of JavaScript tagging
Besides the fact that JavaScript tagging cannot protect you from bots on its own (especially the more sophisticated bots that can more convincingly mimic human behavior), some other challenges they face have to do with privacy and performance:
- Privacy concerns: Collecting and analyzing user behavior can raise privacy concerns. Websites must ensure they comply with privacy regulations like GDPR, which require user consent before tracking certain types of data. Failure to adhere to these rules can result in legal complications.
- Performance overhead: Embedding JavaScript for behavioral analysis can add some overhead to website performance. If not optimized, excessive data collection or complex analysis may slow down the website, and negatively impact user experience.
Finally, you should keep in mind that some bots are programmed to disable JavaScript or execute scripts in a non-interactive manner. As a result, they may bypass JavaScript-based tagging altogether. That’s another reason why you need complementary detection techniques to protect your app and users.
JavaScript tagging as part of a full bot detection solution
JavaScript tagging should be part of a layered defense strategy. While it excels at identifying suspicious activity, it’s not foolproof on its own and may struggle against more sophisticated bots that can mimic human-like behavior more convincingly. Some bots may use machine learning techniques to adjust their behavior to appear more human.
To effectively stop bots, you should combine JavaScript tagging with other methods, such as CAPTCHA, IP reputation checks, rate limiting, device fingerprinting, and behavioral analysis.
If you don’t have the time, knowledge, or resources to implement all these by yourself, you can choose a platform that offers real-time protection against bots, fraud, and abuse, like WorkOS Radar.
Radar tracks user behavior when they sign in to your app. It uses this information to look for any suspicious or unusual activity. If Radar detects something suspicious, it can block or challenge the sign-in based on your settings. Radar also uses a unique method to identify the device by looking at over 20 different characteristics. It tracks every sign-in attempt to spot any that seem odd or impossible. If something unusual happens, Radar alerts you immediately so your admins can take action and notify users if their accounts might be at risk.
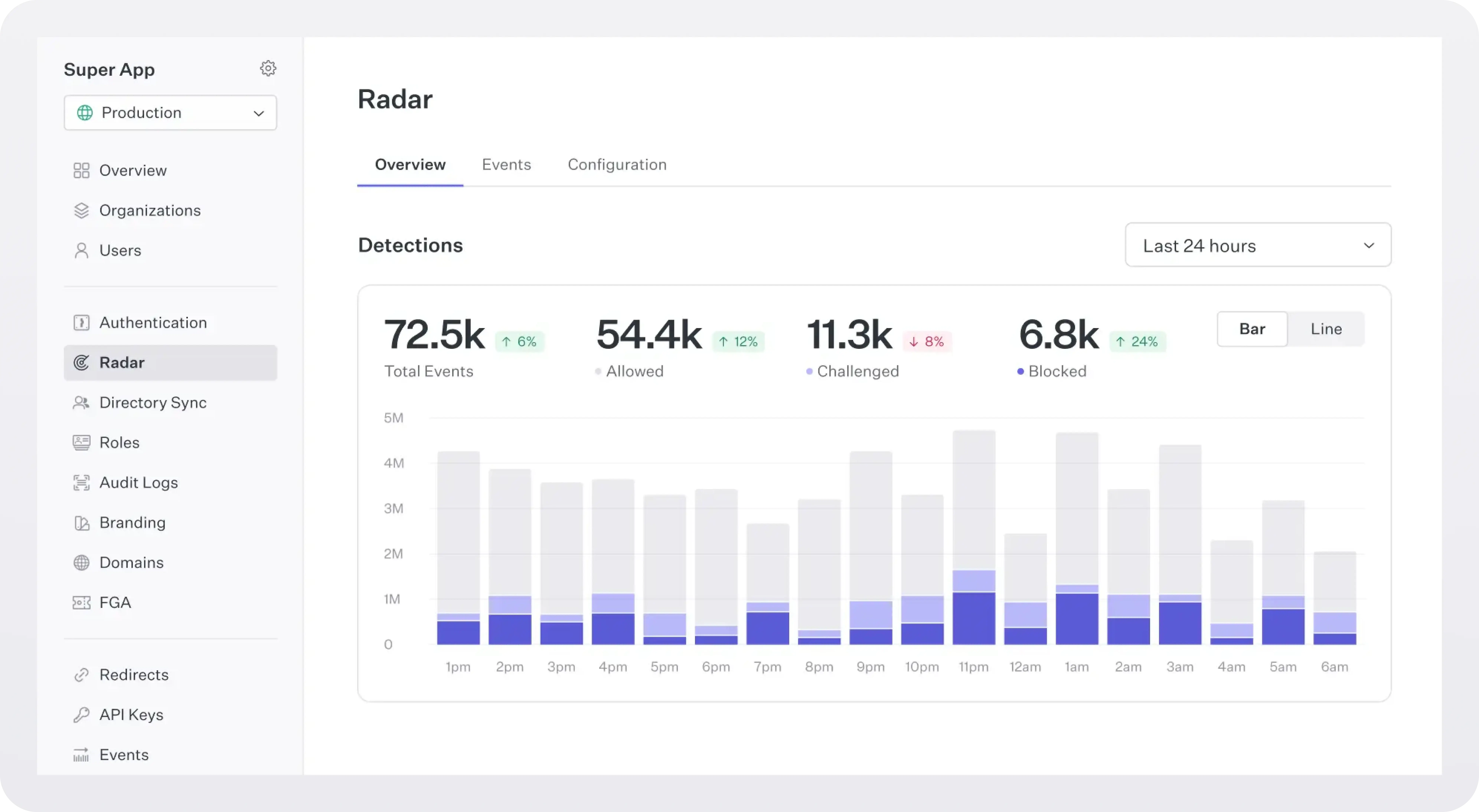
Conclusion
Tracking bot activity with JavaScript tagging is a powerful and effective way to identify malicious users on your site. By monitoring user behavior, tracking mouse movements, analyzing scroll patterns, and even using browser fingerprinting, you can detect and mitigate bots before they cause harm. Implementing these techniques in your web application will help protect your site from scraping, fraud, and other malicious activities.
By combining behavioral analysis with other security measures, you can build a robust defense against automated threats and ensure that your users have a safe and seamless experience.
And if you are ready to step-up your bot detection game, choose a product that specializes in detecting, verifying, and blocking bad actors in real-time. For more on this, see Defending against bad actors: WorkOS Radar vs Castle vs Auth0 vs Stytch vs Arcjet.