How to build a game-building agent system with CrewAI
A hands-on guide with patterns, agents, and executable examples
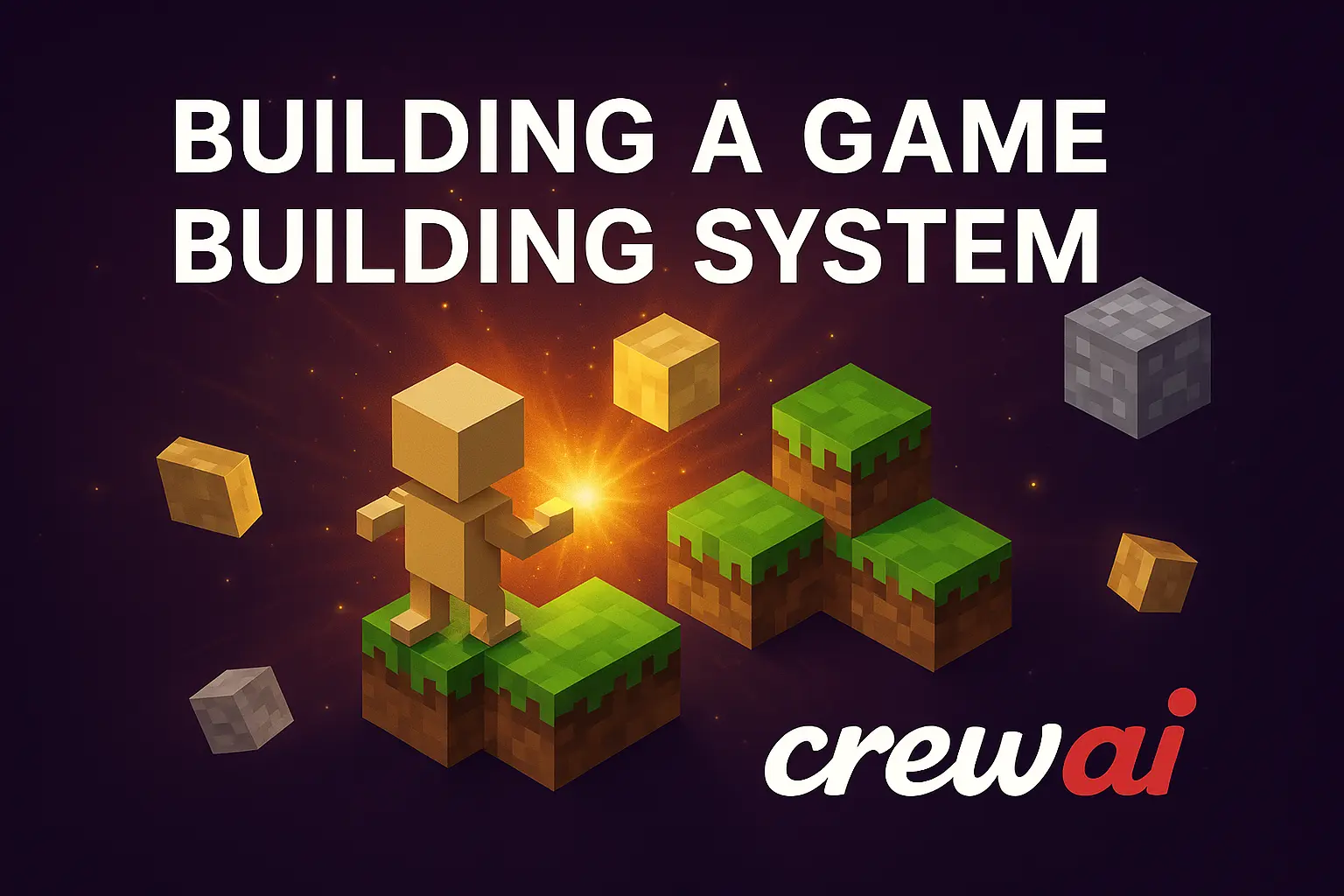
This guide demonstrates how to set up a multi-agent workflow using CrewAI to generate, review, and validate a Python game.
Along the way, we’ll introduce common CrewAI patterns—crews and Flows—to help you structure multi-agent systems with both autonomous collaboration and precise control.
What is CrewAI?
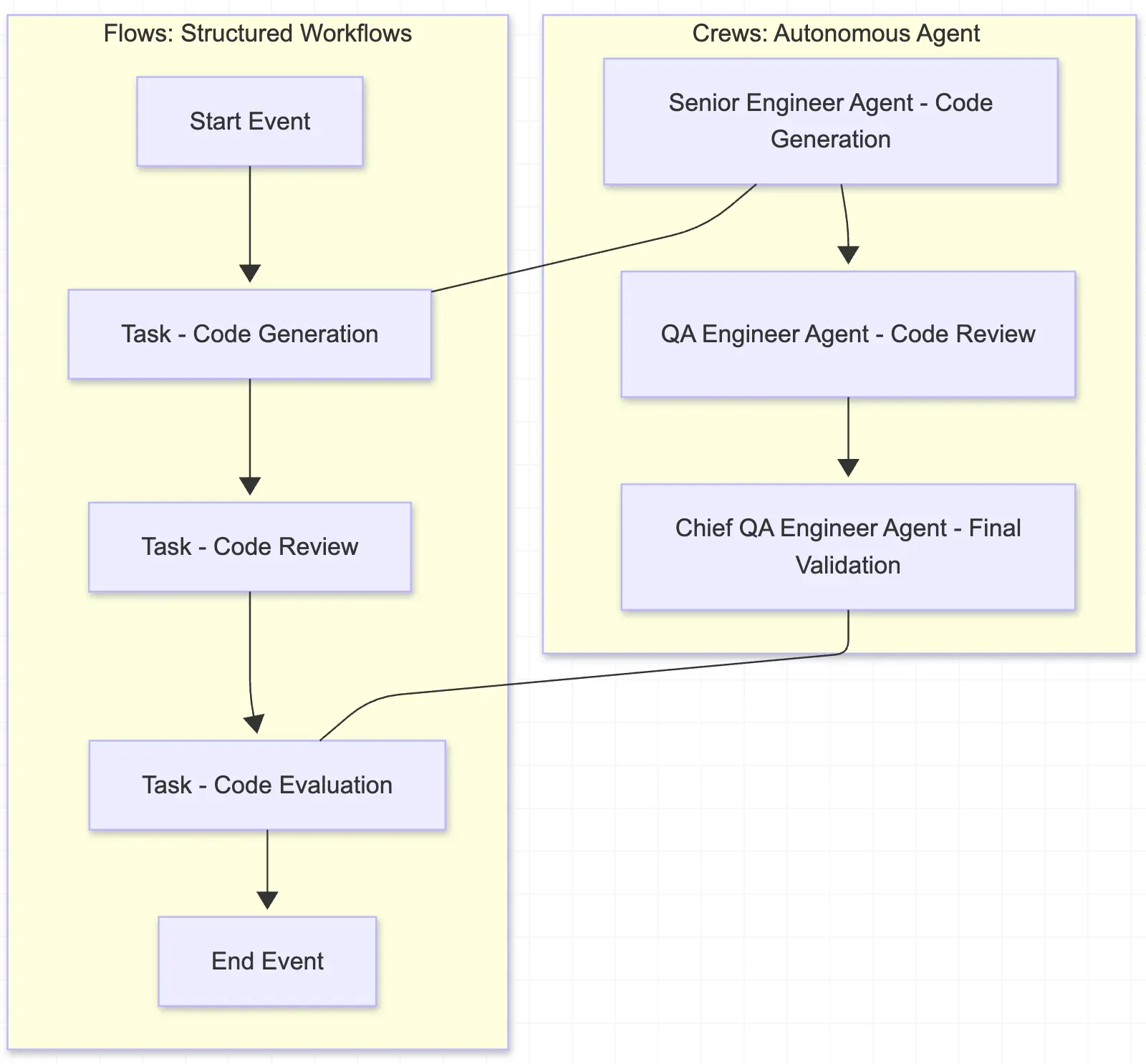
CrewAI is a Python framework that orchestrates multiple AI agents, each dedicated to a specific task. In our example, agents work together to generate a Python game by:
- Producing the initial game code
- Reviewing the code for errors
- Validating that the final output meets the required specifications
At the same time, CrewAI supports two key design patterns:
- Crews: Groups of autonomous agents collaborating on loosely defined tasks.
- Flows: Structured, event-driven workflows that offer granular control over task execution.
This guide uses the game-building example as a hands-on way to learn how these patterns are applied in practice.
Prerequisites
Before getting started, make sure you have:
- Python 3.9+ installed
- Poetry installed (for package management)
- An OpenAI API key (for LLM access)
Environment setup
First, make sure you have Python 3 installed and available:
python3 --version
If it's not installed, download it from python.org.
If you don't have Poetry installed, follow the instructions on the Poetry website.
Create a new project folder and navigate to it:
mkdir crew_ai_gamebuilder cd crew_ai_gamebuilder
Set up a new Poetry project with the required dependencies by creating a pyproject.toml
file:
[tool.poetry]
name = "crew_ai_gamebuilder"
version = "0.1.0"
description = "A CrewAI-based game generator using multiple AI agents"
authors = ["Zack Proser <zack@workos.com>"]
readme = "README.md"
package-mode = false
[tool.poetry.dependencies]
python = ">=3.10,<3.13"
crewai = "^0.108.0"
pyyaml = "^6.0.1"
pygame = "^2.5.2"
langchain = "^0.1.5"
langchain-openai = "^0.0.8"
[build-system]
requires = ["poetry-core"]
build-backend = "poetry.core.masonry.api"
Initialize your Poetry environment and install dependencies:
poetry install
Export your OpenAI API key as an environment variable:
export OPENAI_API_KEY="your-api-key-here"
# On Windows: set OPENAI_API_KEY="your-api-key-here"
Project structure
game_builder_crew/
├── config/
│ ├── agents.yaml
│ ├── tasks.yaml
│ └── gamedesign.yaml
├── crew.py
└── main.py
Getting started with CrewAI: Defining your agents
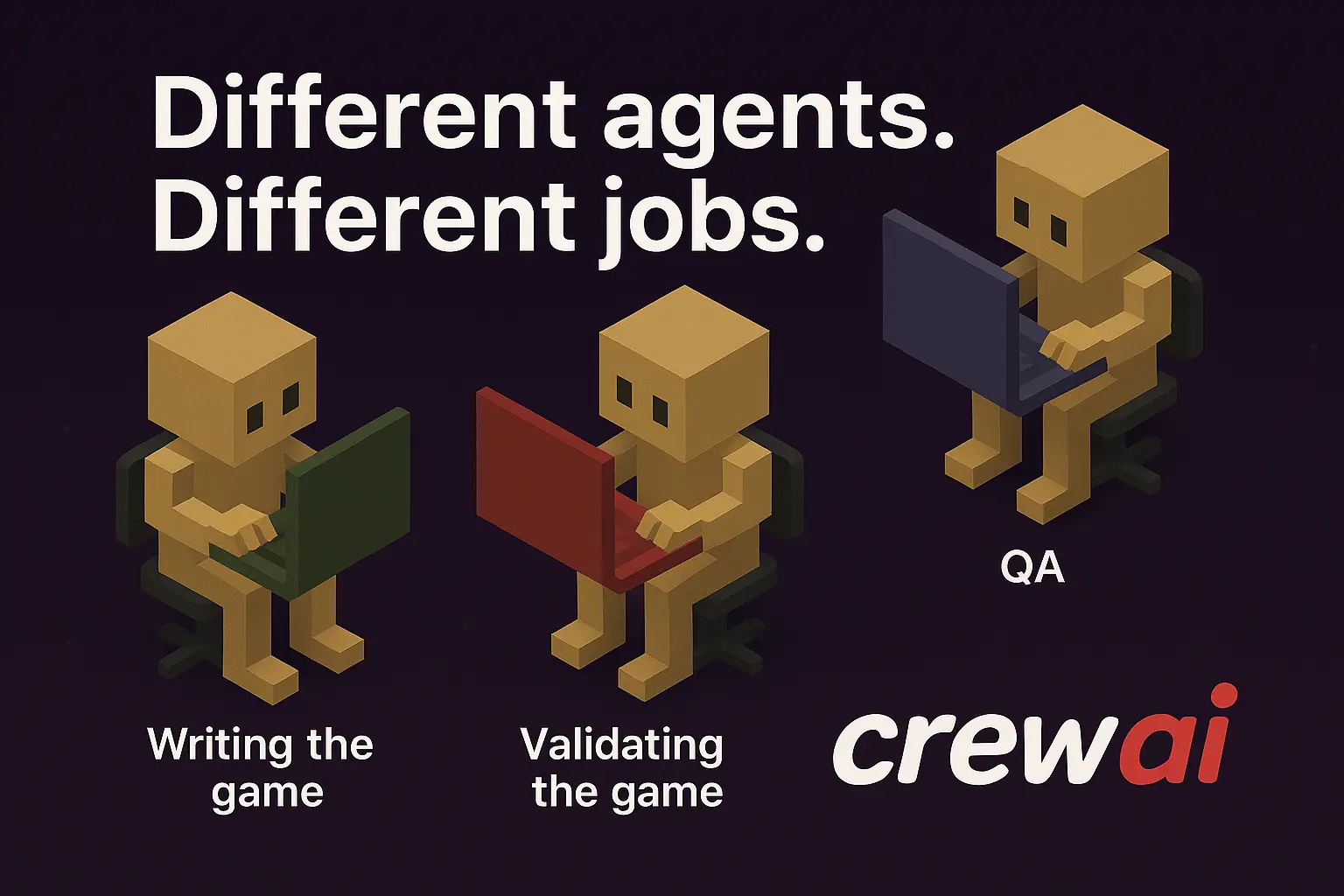
Start by defining your agents and assigning them specific roles. In this example, you’ll configure three agents in your agents.yaml file:
# agents.yaml
senior_engineer_agent:
role: "Senior Software Engineer"
goal: "Write initial Python game code"
backstory: "You are experienced in developing game logic using Python."
qa_engineer_agent:
role: "QA Engineer"
goal: "Review the generated code for syntax and logic errors"
backstory: "Your attention to detail helps you catch subtle bugs and ensure code quality."
chief_qa_engineer_agent:
role: "Chief QA Engineer"
goal: "Perform final validation of the code"
backstory: "You ensure that the final output meets all specified requirements."
Outlining the tasks
Next, define the tasks that each agent will execute in your workflow. In the tasks.yaml file, specify what each task should do and the expected outputs:
# tasks.yaml
code_task:
description: "Generate a complete Python script for the game based on provided game requirements."
expected_output: "Return only the final Python code."
review_task:
description: "Review the generated code for errors, missing imports, and logical issues."
expected_output: "Return the corrected Python code."
evaluate_task:
description: "Ensure the code meets all specified requirements and is production-ready."
expected_output: "Return the final validated Python code."
Each task is designed with precise instructions so that the output of one agent can be passed seamlessly to the next.
Providing game context
Agents need domain-specific context to generate meaningful output. In our example, game design requirements are provided via gamedesign.yaml.
For instance, a simplified Pac-Man game might be described as follows:
# gamedesign.yaml
example1_pacman:
description: |
Create a Pac-Man style game where the player navigates a maze, collects pellets,
and avoids ghosts. Key features include:
- Grid-based movement
- Keyboard arrow key controls
- Scoring: 10 points per pellet, 50 points per power pellet
- Basic ghost AI to chase the player
... additional examples follow ...
When the code generation task runs, the Senior Software Engineer agent uses this context to inform the game logic.
Implementing the GameBuilderCrew class
Let's create the main crew.py
file that defines our GameBuilderCrew
class:
# crew.py
from typing import List
from crewai import Agent, Crew, Process, Task
from crewai.project import CrewBase, agent, crew, task
@CrewBase
class GameBuilderCrew:
"""GameBuilder crew for generating Python games"""
agents_config = 'config/agents.yaml'
tasks_config = 'config/tasks.yaml'
def __init__(self):
super().__init__()
self.game_design = None
def set_game_design(self, game_design):
"""Set the game design that will be used by the agents"""
self.game_design = game_design
@agent
def senior_engineer_agent(self) -> Agent:
config = self.agents_config['senior_engineer_agent']
config['backstory'] += f"\n\nCurrent Game Design Requirements:\n{self.game_design}"
return Agent(
config=config,
allow_delegation=False,
verbose=True
)
@agent
def qa_engineer_agent(self) -> Agent:
config = self.agents_config['qa_engineer_agent']
config['backstory'] += f"\n\nCurrent Game Design Requirements:\n{self.game_design}"
return Agent(
config=config,
allow_delegation=False,
verbose=True
)
@agent
def chief_qa_engineer_agent(self) -> Agent:
config = self.agents_config['chief_qa_engineer_agent']
config['backstory'] += f"\n\nCurrent Game Design Requirements:\n{self.game_design}"
return Agent(
config=config,
allow_delegation=True,
verbose=True
)
@task
def code_task(self) -> Task:
return Task(
config=self.tasks_config['code_task'],
agent=self.senior_engineer_agent()
)
@task
def review_task(self) -> Task:
return Task(
config=self.tasks_config['review_task'],
agent=self.qa_engineer_agent(),
context=[self.code_task()]
)
@task
def evaluate_task(self) -> Task:
return Task(
config=self.tasks_config['evaluate_task'],
agent=self.chief_qa_engineer_agent(),
context=[self.review_task()]
)
@crew
def crew(self) -> Crew:
"""Creates the GameBuilderCrew"""
return Crew(
agents=self.agents, # Automatically created by the @agent decorator
tasks=self.tasks, # Automatically created by the @task decorator
process=Process.sequential,
verbose=True
)
This kickoff
method:
- Initializes the Crew from preloaded agent and task configs
- Injects the game spec from YAML as dynamic input
- Runs all agents in sequence: code → review → validate
- Returns the final game code
Orchestrating a Crew with Python
Now, let's create the main script to run our game builder crew:
# main.py
import yaml
import sys
from crew import GameBuilderCrew
def list_available_games(examples):
"""Display available game examples"""
print("\nAvailable games:")
for i, game_name in enumerate(examples.keys(), 1):
print(f"{i}. {game_name}")
return list(examples.keys())
def select_game(examples):
"""Let user select which game to generate"""
games = list_available_games(examples)
while True:
try:
choice = input("\nSelect a game to generate (number): ")
index = int(choice) - 1
if 0 <= index < len(games):
return examples[games[index]]
print("Invalid selection. Please try again.")
except ValueError:
print("Please enter a number.")
def run():
print("## Welcome to the Game Crew")
print('-------------------------------')
# Load game design examples
with open('config/gamedesign.yaml', 'r', encoding='utf-8') as file:
examples = yaml.safe_load(file)
# Let user select which game to generate
selected_game = select_game(examples)
# Create crew instance and set the game design
crew = GameBuilderCrew()
crew.set_game_design(selected_game)
# Run the crew
result = crew.crew().kickoff()
print("\n\n########################")
print("## Here is the result")
print("########################\n")
print(result)
# Save the code to a file
with open('game.py', 'w') as f:
f.write(str(result))
print("\nCode has been saved to game.py")
print("\nTo run the game, use:")
print("poetry run python3 game.py")
if __name__ == "__main__":
run()
Understanding the patterns: crews vs. flows
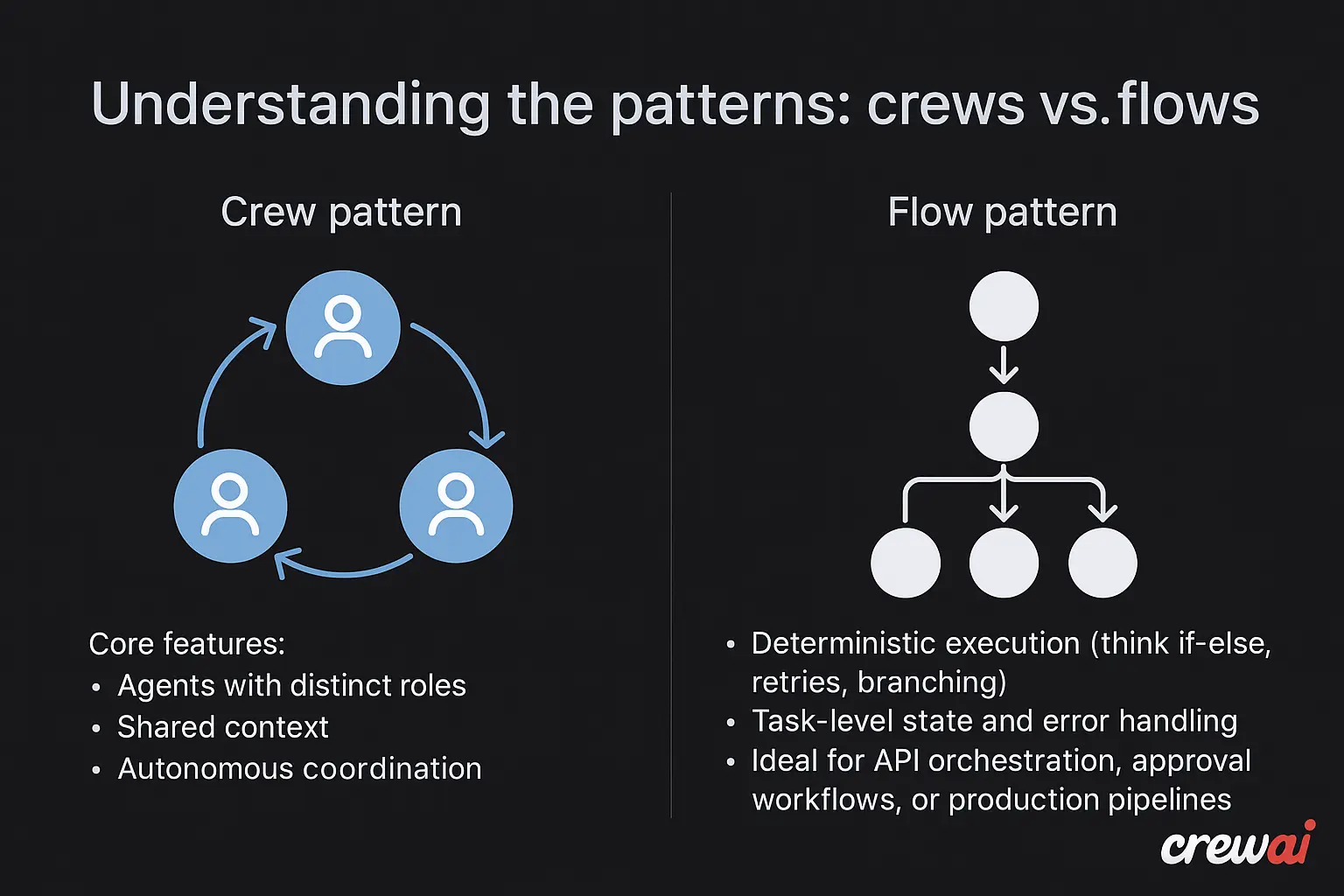
Understanding the patterns: Crews vs. Flows
This example uses the Crew pattern with a sequential process (Process.sequential
), which is well-suited for collaborative work that needs to follow a specific order. The key characteristics are:
- Agents with distinct roles
- Shared context passing between tasks
- Sequential task execution where outputs from one task feed into the next
The example implementation explicitly uses process=Process.sequential
In the Crew definition, tasks are executed one after another in a predefined order, with each task waiting for the previous one to complete.
The Flow pattern is a more advanced pattern that offers even greater structure and control:
- Deterministic execution with conditional branching
- Task-level state and error handling
- Fine-grained control over task execution sequence
Hybrid approaches are also supported. For example, you could use sequential processing for main tasks while allowing hierarchical delegation within certain agent interactions.
This pattern isn't just for games. You can use the same approach to:
- Automate blog writing with SEO review and copy editing
- Create product spec generators with legal and engineering validation
- Build internal documentation bots that research, write, and fact-check
Anywhere you need modular, role-based intelligence with sequential validation steps, the crew architecture pattern fits perfectly.
Let's build a game!
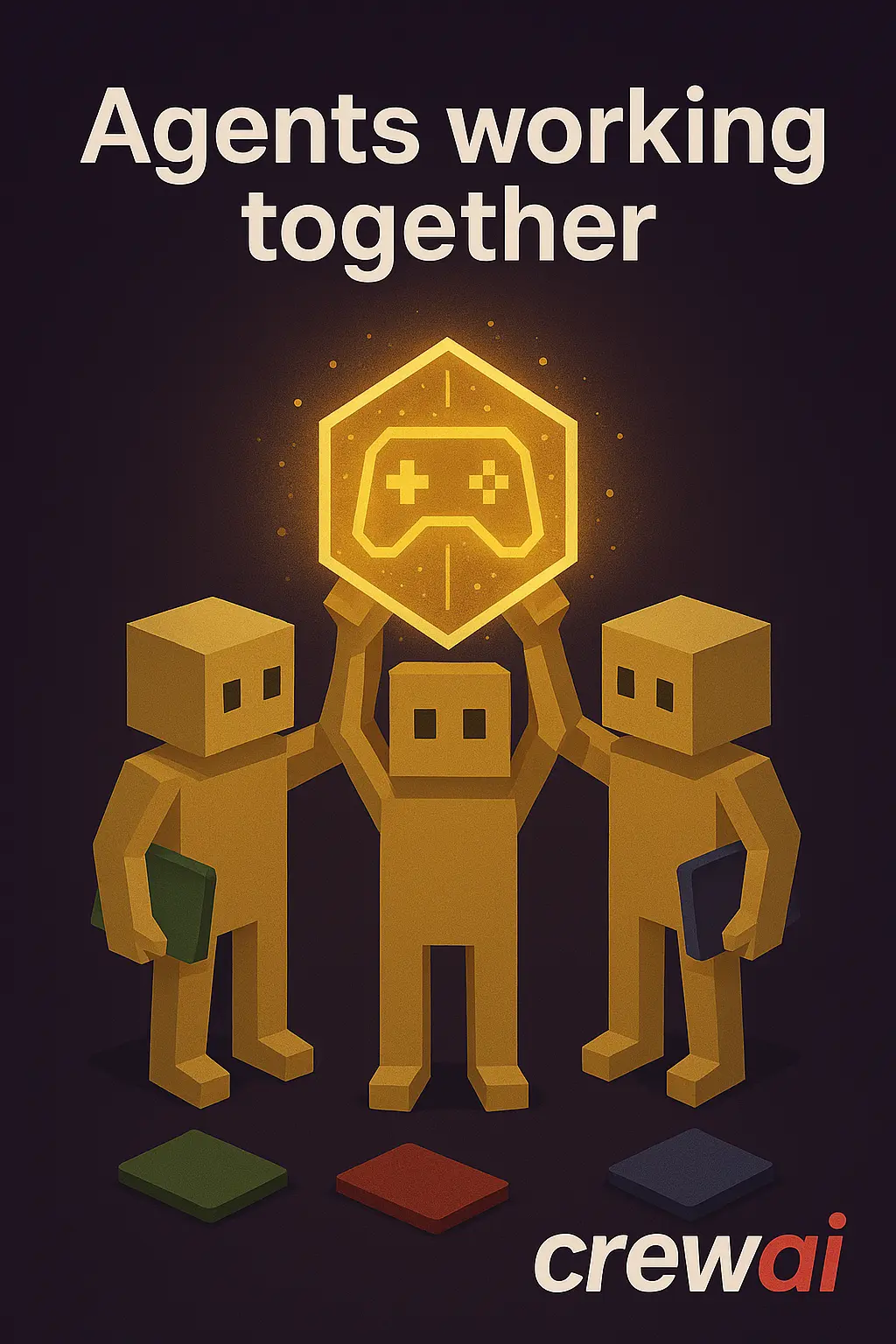
Run the game builder crew
To run the game builder with Poetry, execute:
poetry run python3 main.py
You’ll see the various agents pick up work and pass results between themselves:
─────────────────────────────────────── Crew Execution Started ───────────────────────────────────────╮
│ │
│ Crew Execution Started │
│ Name: crew │
│ ID: df8756b6-da0b-4ca2-b500-c689dd253c65 │
│ │
│ │
╰──────────────────────────────────────────────────────────────────────────────────────────────────────╯
🚀 Crew: crew
└── 📋 Task: 5627efb4-cc4a-4f44-b5f6-eec2bdf64dfb
Status: Executing Task...
🚀 Crew: crew
└── 📋 Task: 5627efb4-cc4a-4f44-b5f6-eec2bdf64dfb
Status: Executing Task...
└── 🤖 Agent: Senior Software Engineer
Status: In Progress
# Agent: Senior Software Engineer
## Task: Generate a complete Python script for the game based on provided game requirements.
🚀 Crew: crew
└── 📋 Task: 5627efb4-cc4a-4f44-b5f6-eec2bdf64dfb
Status: Executing Task...
└── 🤖 Agent: Senior Software Engineer
Status: In Progress
└── 🧠 Thinking...Running the Game Builder
After describing a Pong-like game, this author was able to run the finished product of this crew immediately:

You can also paste the final game output into Replit and see that it creates a working game:

Final thoughts
By defining a small crew of agents, assigning each one a role and task, and wiring everything together with Python, you’ve built a game-generating pipeline powered by AI agents.
Along the way, you also saw how the Crew and Flow patterns differ—and how they can be combined for hybrid use cases.To experiment further, visit CrewAI on GitHub and try modifying the YAML or agent logic for your own workflows.