Fine-Grained Authorization
Flexible? Fast? Scalable? Check. Check. Check.
Granular access control and fast authorization checks at scale. Build and deploy modern apps with a fully managed FGA solution.
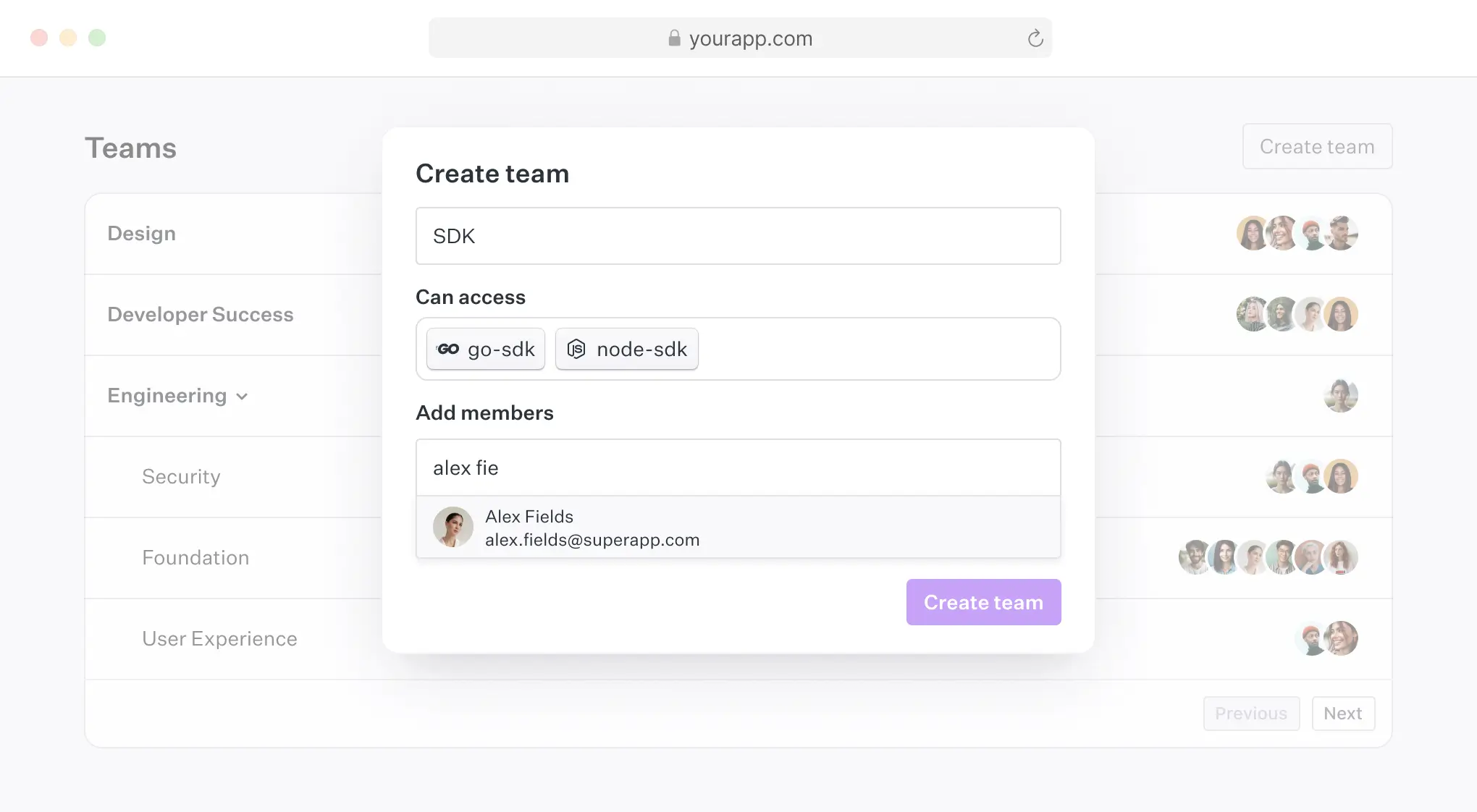



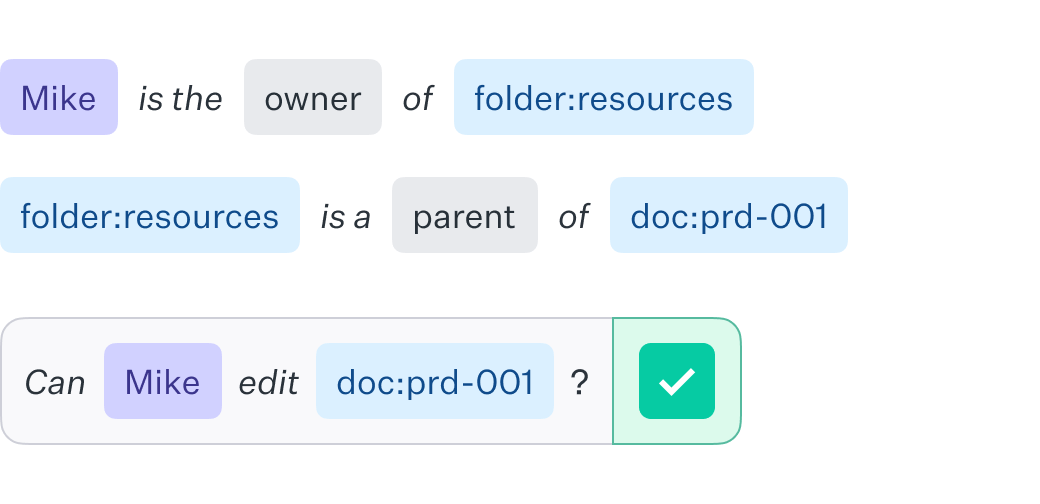

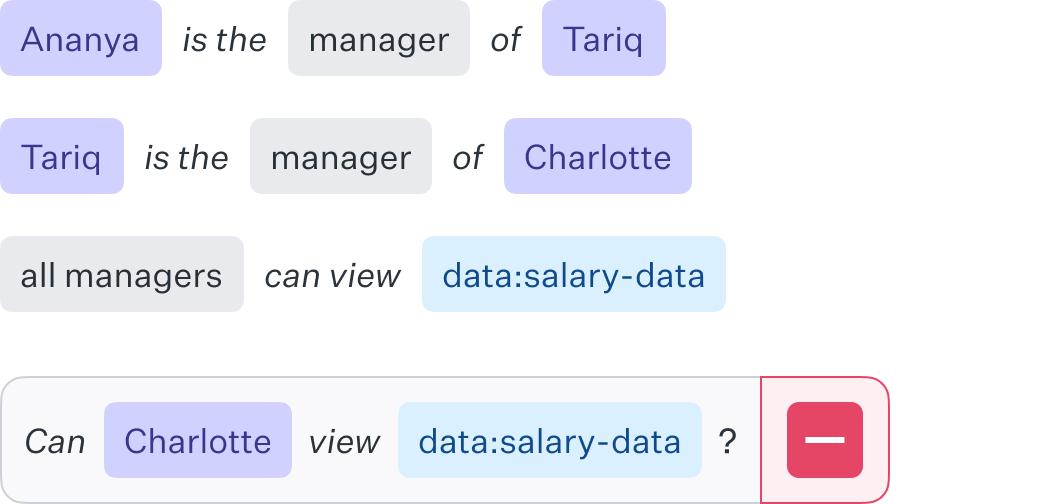



One system. Millions of uses.
Define precise access controls that go beyond RBAC. Implement fine-grained permissions in a single, flexible system.
Future-proof your permissions model.
Say goodbye to refactoring permissions. WorkOS FGA enables you to accelerate development by decoupling permissions from your core application logic.
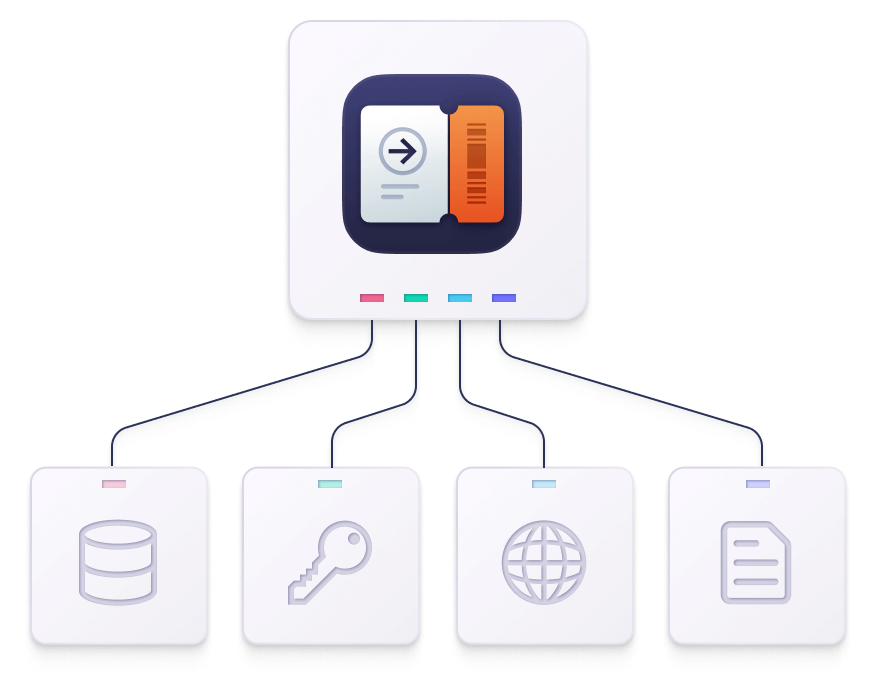
Monoliths to microservices, FGA works everywhere.
A centralized, single source of truth for your permissions that scales with your growth.
Running globally at the speed of light.
Easily handle high traffic permissions checks with minimal latency. Guaranteed reliability backed by multi-region redundancy and elastic auto-scaling.
Add fine-grained authorization to any application.
Decoupled authorization
Replace complex, conditional authorization logic with simple REST APIs.Authorization as schema
Build any authorization model with a simple schema language.Fast runtime APIs
Performance-optimized check and query APIs to enable fast permissions checks.
Go beyond RBAC
Express any authorization model including RBAC, ReBAC, ABAC, organization hierarchies, and entitlements with a simple schema language.
Debug and audit everything
All system operations are automatically logged for easy, real-time permissions audits and debugging.
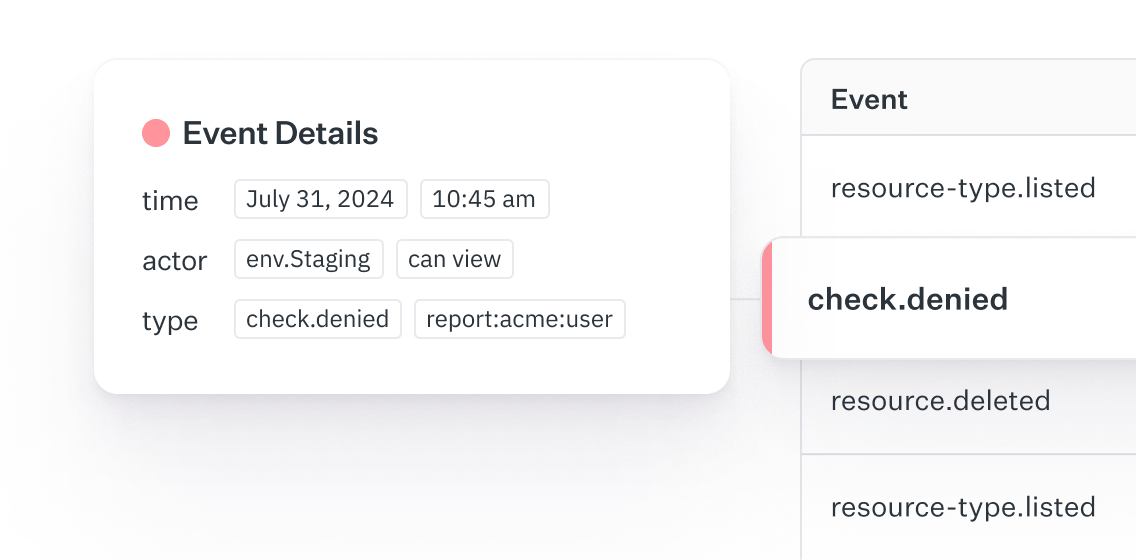
Secure, out of the box
We check all the boxes so you can focus on what you do best, building your app and serving your customers.
Get started today, for free
Add FGA to your application today.
The free tier includes 10M operations per month.