Prisma ORM for TypeScript - A technical primer
Prisma is one of the most popular Object-Relational Mappers (ORMs) in the TypeScript/JavaScript ecosystem due to its robust type-safety guarantees and seamless integration with frameworks like Next.js.
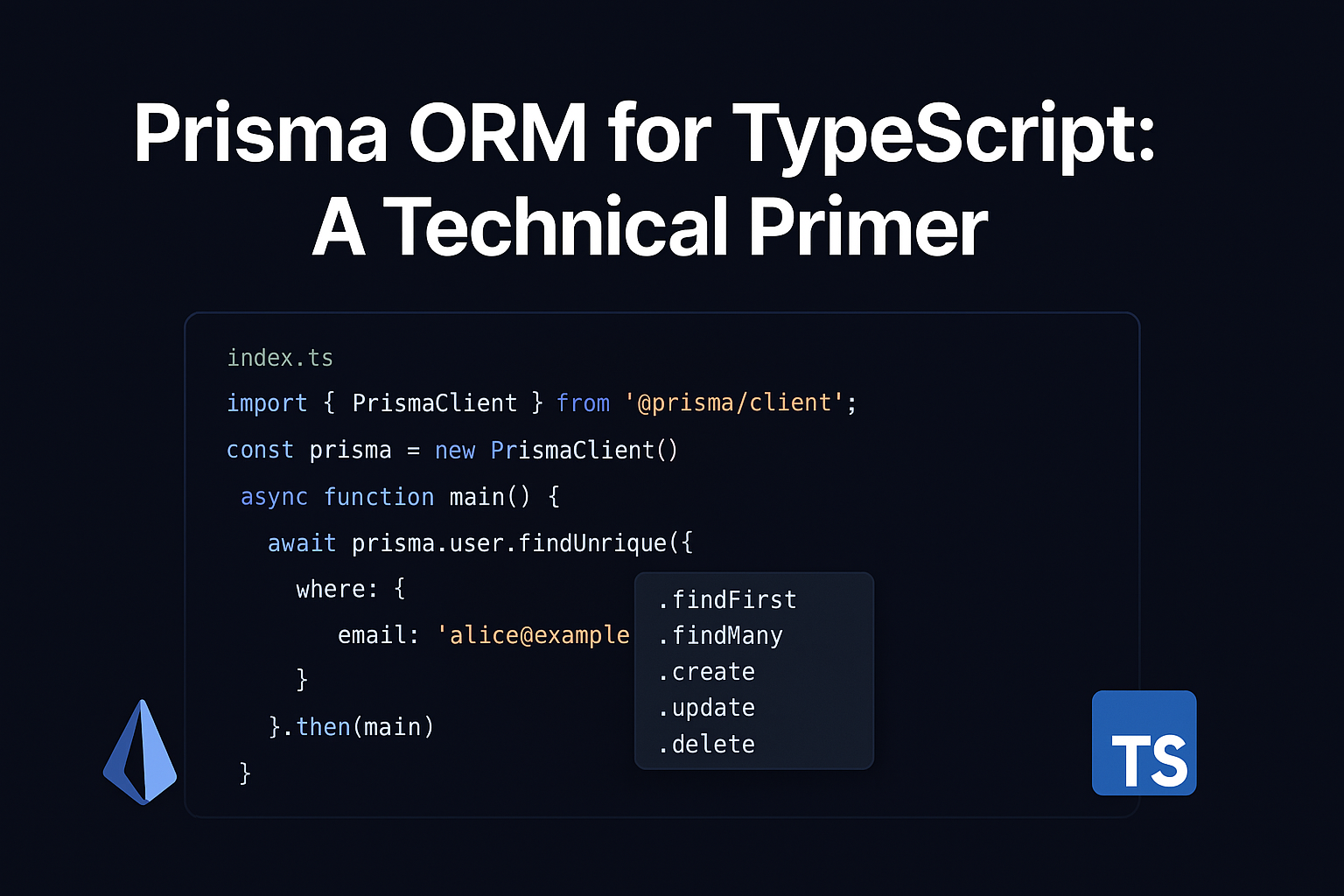
Modern web development teams prefer type-safe solutions for streamlined development and lower maintenance overhead.
Prisma offers powerful, auto-generated type definitions and a consistent API for database operations.
What is Prisma?
Prisma is a TypeScript ORM that focuses on:
- Zero-cost type-safety: It automatically generates TypeScript types based on your schema, helping catch potential query errors at compile-time.
- Intuitive data modeling: You define your data model via the prisma.schema file using Prisma’s modeling language, and Prisma handles the rest (including migrations, queries, and relations).
- High performance: It features built-in connection pooling, optimized queries, and a built-in DataLoader to avoid the notorious “N+1 query problem.”
Prisma works with relational databases like PostgreSQL, MySQL, MariaDB, SQLite, and SQL Server, making it suitable for many production environments.
Why Developers Like Prisma + TypeScript
Type-Safe queries
Prisma automatically infers return types for each query, guaranteeing correctness whenever you fetch or manipulate data. This reduces runtime errors and clarifies the exact shape of the response at a glance.
Developer experience & autocompletion
Prisma comes with a code generator that creates a fully typed client. In TypeScript-friendly IDEs, you’ll see instant IntelliSense autocompletion for fields, filters, relations, and so on, which accelerates coding and reduces mistakes.
Easy migrations
Instead of manually writing SQL migrations, you modify your prisma schema file and run prisma migrate dev (or Prisma migrate deploy). Prisma generates SQL migrations under the hood, simplifying your schema changes significantly.
Fits naturally with Next.js
Next.js’ full-stack capabilities let you place Prisma calls in API routes or server-side code. This approach keeps sensitive database logic off the client and coordinates easily with Next.js’ SSR or server actions (in Next.js 13+).
Reduced boilerplate
Creating relationships (1:1, 1:n, n:n) is as simple as adding relation fields in your schema. Prisma then handles all the tricky join logic behind the scenes, letting you fetch related data through an intuitive API.
Potential downsides and considerations
Learning curve
While Prisma is well-documented, teams new to TypeScript or ORMs may need to adjust to its declarative schema and migration workflow.
Abstraction over complex queries
Many advanced database-specific features (like complex window functions or certain raw queries) might need direct SQL usage (which Prisma does allow through prisma.$queryRaw). Still, it’s not always as straightforward as writing native SQL from the start.
Extra build step
Each time you modify your schema, you’ll need to generate the latest Prisma Client. This typically isn’t onerous, but is one more step in your CI/CD pipeline.
Lock-in
As with any ORM, you rely on Prisma’s query-building and migration system. Some teams prefer direct SQL or a more minimal query builder like Knex to maintain database “portability.” For many, though, Prisma’s abstraction is a net positive.
Basic example in Next.js
Below is a quick sketch of how you might incorporate Prisma into a Next.js project. (Assumes you’ve run npm install prisma @prisma/client or yarn add prisma @prisma/client and set up your prisma.schema.)
/prisma/schema.prisma (Minimal Example)
The schema file sets up the connection to your database as well as the models.
datasource db {
provider = "postgresql"
url = env("DATABASE_URL")
}
generator client {
provider = "prisma-client-js"
}
model User {
id String @id @default(uuid())
email String @unique
name String
posts Post[]
}
model Post {
id String @id @default(uuid())
title String
createdAt DateTime @default(now())
userId String
user User @relation(fields: [userId], references: [id])
}
/lib/prisma.ts (Prisma Client Singleton)
Here’s how you expose the Prisma Client as a singleton that can be loaded throughout your application’s API routes and pages as needed:
import { PrismaClient } from '@prisma/client';
const globalForPrisma = global as unknown as { prisma: PrismaClient };
export const prisma =
globalForPrisma.prisma ||
new PrismaClient({
// optional log settings, etc.
});
if (process.env.NODE_ENV !== 'production') {
globalForPrisma.prisma = prisma;
}
/pages/api/users.ts (Example Next.js API route)
Here’s an example of how you’d use the Prisma client in an API route:
import type { NextApiRequest, NextApiResponse } from 'next';
import { prisma } from '../../lib/prisma';
export default async function handler(req: NextApiRequest, res: NextApiResponse) {
if (req.method === 'GET') {
const users = await prisma.user.findMany({
include: { posts: true },
});
return res.status(200).json(users);
}
if (req.method === 'POST') {
const { email, name } = JSON.parse(req.body);
const newUser = await prisma.user.create({
data: {
email,
name,
},
});
return res.status(201).json(newUser);
}
return res.status(405).send('Method not allowed.');
}
Final thoughts
Prisma aligns perfectly with TypeScript-centric tech stacks by providing:
- Automatic type generation for all models and queries
- Streamlined migrations that eliminate repetitive SQL
- Developer-focused ergonomics (autocomplete and intuitive schema definitions)
While there is a learning curve and certain edge cases that may require raw SQL, Prisma is a powerful, battle-tested ORM that speeds up development and reduces errors.
Modern frameworks such as Next.js integrate easily with it, producing a developer experience that feels cohesive and maintainable.
Prisma is well worth considering for its type safety, automated workflows, and seamless Next.js compatibility.
References & further reading:
- Prisma Documentation – Official guides on schema, migrations, and integration examples.
- Prisma & TypeScript Official Page – Overview of how Prisma and TypeScript fit together.
- Next.js Docs – Official Next.js documentation for server routes, environment variables, etc.