Top Ruby gems for authentication & authorization
Looking to secure your Ruby on Rails app? Discover the top gems for authentication and authorization that will protect your users. From seamless sign-ins to granular user permissions, these gems have got you covered.
When it comes to authentication and authorization, there are several Ruby gems that stand out due to their ease of use, security features, and community support.
In this article, we will review some of the top gems for both authentication and authorization:
- Authentication gems:
- Authorization gems:
Devise: Feature-rich and powerful
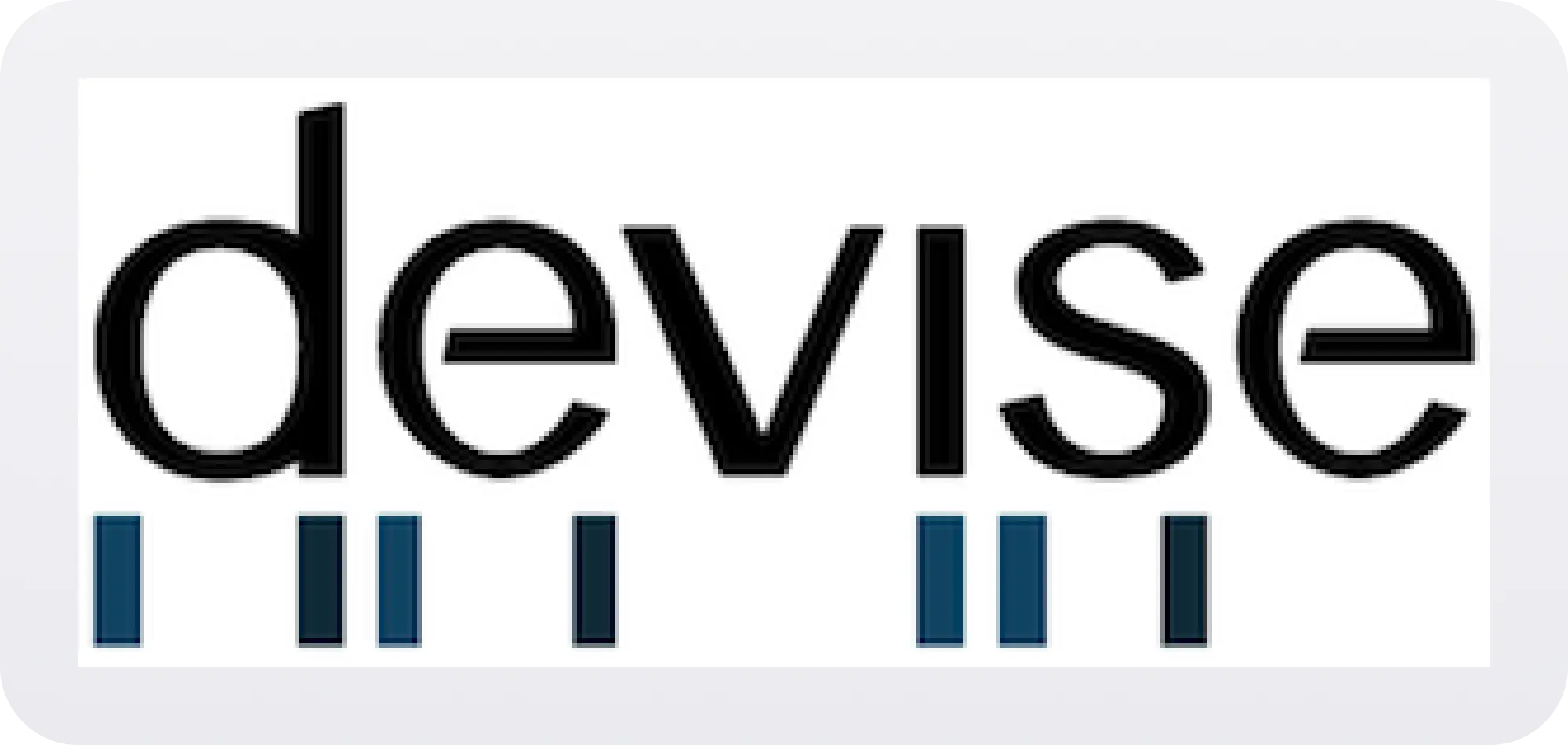
Devise stands out as the most comprehensive authentication solution for Rails, offering a wide range of built-in modules for common authentication tasks. Unlike other gems, Devise provides features like database-backed authentication, account locking, email confirmation, password recovery, session management, and OmniAuth support for third-party logins out of the box.
Devise is composed of 10 modules, and you can choose which ones you want to use:
- Database Authenticatable: This is the core authentication module. It authenticates users by comparing the password provided with the one stored in the database (hashed). This module automatically manages user sessions and ensures secure login. The authentication can be done both through POST requests or HTTP Basic Authentication.
- Omniauthable: This module allows users to authenticate using external authentication providers like Google, Facebook, GitHub, etc., using OmniAuth.
- Confirmable: This module sends a confirmation email to users when they register, requiring them to verify their email address before fully activating their account. This is useful for ensuring the validity of the user's email (a best practice you should be following to avoid vulnerabilities).
- Recoverable: This module sends a password reset link to the user's registered email so they can reset their passwords if they forget them.
- Registerable: Manages user sign-up, registration, and account creation, editing, and deletion.
- Rememberable: Implements "remember me" functionality, allowing users to stay logged in across sessions without needing to re-enter their credentials. This works by setting a persistent cookie in the user's browser.
- Trackable: Tracks user sign-in details like the number of sign-ins, IP address, and the timestamp of the last sign-in. This module is useful for monitoring login patterns and user activity and can be used for auditing and security purposes.
- Timeoutable: This module automatically logs users out after a certain period of inactivity. It ensures that inactive sessions are terminated and helps mitigate session hijacking risks.
- Validatable: Adds basic email and password validation for the user model. This module enforces rules like valid email format and password length but can be customized, so you can define your own validations.
- Lockable: Provides functionality to lock user accounts after a set number of failed sign-in attempts. This module helps protect against brute-force attacks by limiting login attempts. An account can unlock via email or after a specified time period.
Devise is one of the most widely used and powerful authentication gems in the Rails ecosystem. It provides everything you need to implement a secure and flexible authentication system. It has excellent documentation and a large community of contributors, making it easy to find help and resources. On the downside, it has a learning curve, it’s an overkill for simple apps, and is primarily built for Rails, so if you are using another Ruby framework, you may need to explore alternatives or integrate Devise manually.
OmniAuth: Authenticate with 3rd party services

OmniAuth is a flexible authentication system that allows you to integrate third-party authentication providers with ease. Whether it's social media logins like Facebook or Twitter, or identity providers like Google, GitHub, or even LinkedIn, OmniAuth supports all of them through simple configurations.
OmniAuth uses the concept of strategies. A strategy is a class that handles the specific logic for authenticating users via a particular third-party service (e.g., OmniAuth::Strategies::Google
for Google). Each OmniAuth strategy implements the authentication flow for a different provider, allowing your application to interface with that provider and securely authenticate users.
Any developer can create strategies for OmniAuth that can authenticate users via disparate systems. In order to use OmniAuth in your applications, you will need to leverage one or more strategies. These strategies are generally released individually as RubyGems, and you can find them in this community maintained list. Some of the most popular are:
- omniauth-google-oauth2
- omniauth-facebook
- omniauth-github
- omniauth-linkedin-oauth2
- omniauth-slack
- omniauth-apple
- omniauth-spotify
There is also an OmniAuth strategy for authenticating with WorkOS: omniauth-workos.
OmniAuth provides a common interface for all authentication providers, meaning that switching or adding new providers doesn't require rewriting authentication logic. This uniformity makes it easy to maintain and extend your authentication flow.
To initialize a strategy, you use OmniAuth::Builder
, specifying the authentication provider and any required keys (such as API keys and secrets).
When the user chooses to authenticate with a provider (e.g., "Sign in with Google"), OmniAuth sends them to the provider’s authentication page.
After the user logs in, the provider redirects them back to your app. OmniAuth will process the callback, extract the necessary information (like the user's name and email), and make it available to your application.
OmniAuth supports customizable callbacks, allowing developers to control what happens after the user successfully authenticates with a third-party service. You can store user details, create accounts, or link existing users based on the authentication data provided by the external service.
Based on the data returned from the provider, you can create or link a user account in your database.
OmniAuth strategies are the backbone of OmniAuth’s ability to simplify third-party authentication. With pre-built strategies for popular services and the ability to create custom ones, OmniAuth provides a powerful, extensible framework for adding authentication to your Rails applications. Whether you want social logins, enterprise integrations, or custom solutions, OmniAuth strategies handle the heavy lifting.
Clearance: Minimalist authentication
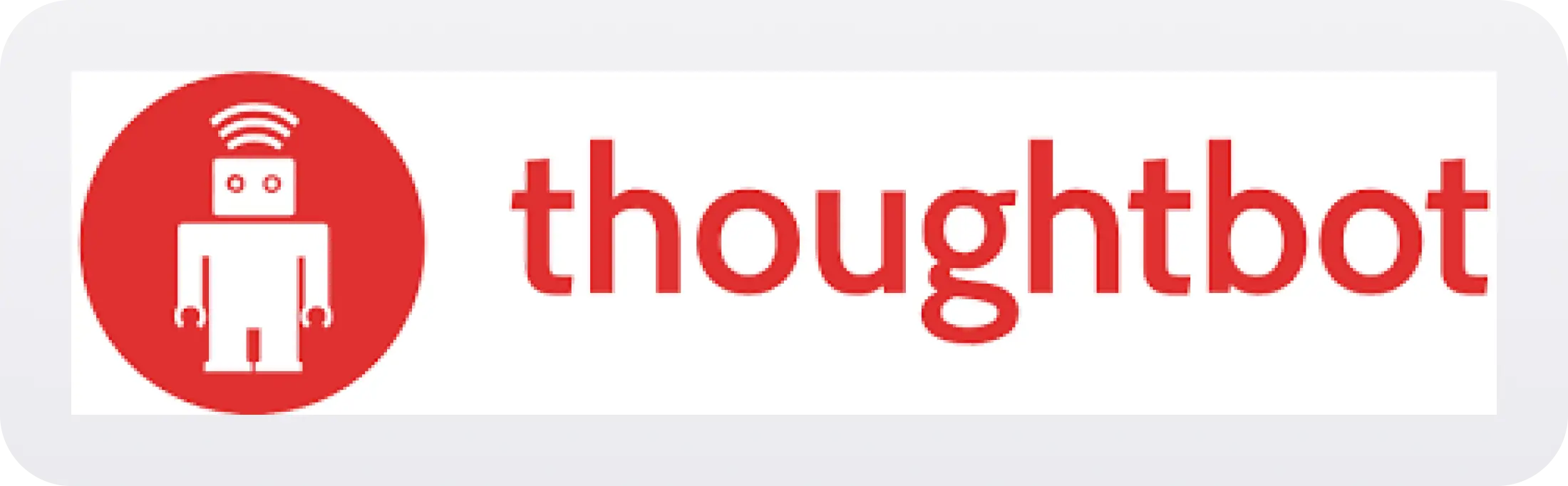
Clearance is a simple and lightweight authentication gem for Rails apps that’s built with a clear aim: to give you just the essentials for handling user sign-ups, logins, and password management. It’s especially useful for developers who want a straightforward, easy-to-implement authentication solution without extra features they don’t need.
One of Clearance’s key selling points is its minimal configuration. You can get started with minimal setup, making it ideal for developers who want a quick, easy way to add authentication without extra overhead. It’s a gem built with Rails in mind, following the Rails conventions and using Rails' built-in ActiveRecord models and features.
Clearance offers the following functionality:
- Sign up and sign in.
- Password reset via email.
- Password hashing.
- You can configure Clearance to require email confirmation after sign-up, ensuring users verify their email addresses before they can fully access your application. This is optional but a best practice that you should follow.
- Session management for logged-in users.
Unlike some other gems (like Devise), Clearance doesn’t have external dependencies or require integrations with third-party services, making it a lighter choice for authentication. It’s ideal for applications that don't require complex features like two-factor authentication, or multiple authentication strategies.
Authlogic: Flexible and customizable
Authlogic is another popular authentication solution for Ruby on Rails that provides a clean, flexible, and modular approach to user authentication. It’s a simpler alternative to Devise, offering just the basics needed for authentication.
Authlogic is often chosen for projects that require a more customizable solution for authentication and prefer a more manual approach compared to solutions like Devise or Clearance. It does not have as many built-in features as Devise, but its simplicity and extensibility are its key selling points.
Some of its key features are:
- Flexible session management system that is easy to use and customize, letting you decide exactly how you want to handle user logins and sessions.
- It doesn’t assume any specific user model structure, allowing you to design your user model (and associated logic) to fit your needs.
- Secure password hashing (typically with bcrypt or SHA1) to protect user passwords.
- You can implement password reset functionality using the token-based system or integrate it with your own custom logic.
- Authlogic supports persistent sessions (i.e., "remember me" functionality) so users stay logged in across sessions, with flexible expiration settings.
- Integrates seamlessly into Rails applications, adhering to Rails conventions and making it easy to add to your project without much setup.
- Allows multiple concurrent sessions per user, so a user can be logged in from multiple devices at the same time (if desired).
- You can extend Authlogic by adding your own custom features, validation, or logic as needed. It is very flexible and doesn’t impose many restrictions.
Authlogic is a good choice for developers who need a flexible, customizable authentication system without the overhead of more feature-rich gems like Devise. It's ideal for applications that require straightforward user authentication, session management, and password handling but don't need additional features like two-factor authentication or social login (which you would need to implement them yourself or integrate other gems). It requires more manual setup and configuration, which may not be ideal for those looking for a plug-and-play solution, but it's a solid option for Rails developers seeking a tailored authentication solution.
Rodauth: A feature-rich authentication framework for Rails and Rack
Rodauth is a comprehensive and flexible authentication library designed for Rack-based Ruby applications, with a focus on security and performance. It is well-suited for use with Ruby on Rails but is framework-agnostic, meaning it can be integrated with any Rack-based framework like Sinatra, Padrino, or even custom Rack applications.
Rodauth allows developers to build sophisticated authentication systems with features like multi-factor authentication, account locking, password resets, and much more, all while maintaining a high level of flexibility and configurability.
Some of its key features include:
- Rodauth offers a complete authentication framework, including login, registration, password management (reset, change), email verification, and more, all out of the box.
- Built-in support for two-factor authentication (2FA) via email or SMS.
- Rate-limiting on sensitive actions, like login attempts and account locking after multiple failed login attempts. Users can unlock their accounts through secure methods.
- Support for external authentication providers like Google, Facebook, or GitHub.
- Allows you to enforce password complexity rules (such as minimum length or special characters).
- Efficient session management and flexible mechanisms for token-based authentication, making it well-suited for APIs or single-page applications (SPAs).
- Built-in email verification ensures that users' email addresses are valid before they can log in. It also supports sending verification codes via SMS.
In the configuration block, you can enable and configure Rodauth’s many modules, such as login, logout, password reset, and more.
Example of a basic configuration for login and password reset:
Rodauth is an excellent choice for developers who need a highly flexible and feature-rich authentication system. Its security features and customizable nature make it ideal for applications that require advanced authentication workflows. However, it may have a steeper learning curve compared to simpler authentication solutions like Devise, and it’s more suited for developers comfortable with Sequel or those who need fine control over their authentication logic.
If you're building an application where security and customization are key, and you're willing to invest time into learning the system, Rodauth could be the right choice for your needs.
Sorcery: Essential lightweight authentication
!!Sorcery is currently unmaintained. If you decide to use it, you accept the risk that it may break or have unpatched security vulnerabilities. We recommend using one of the alternative libraries that are actively maintained instead.!!
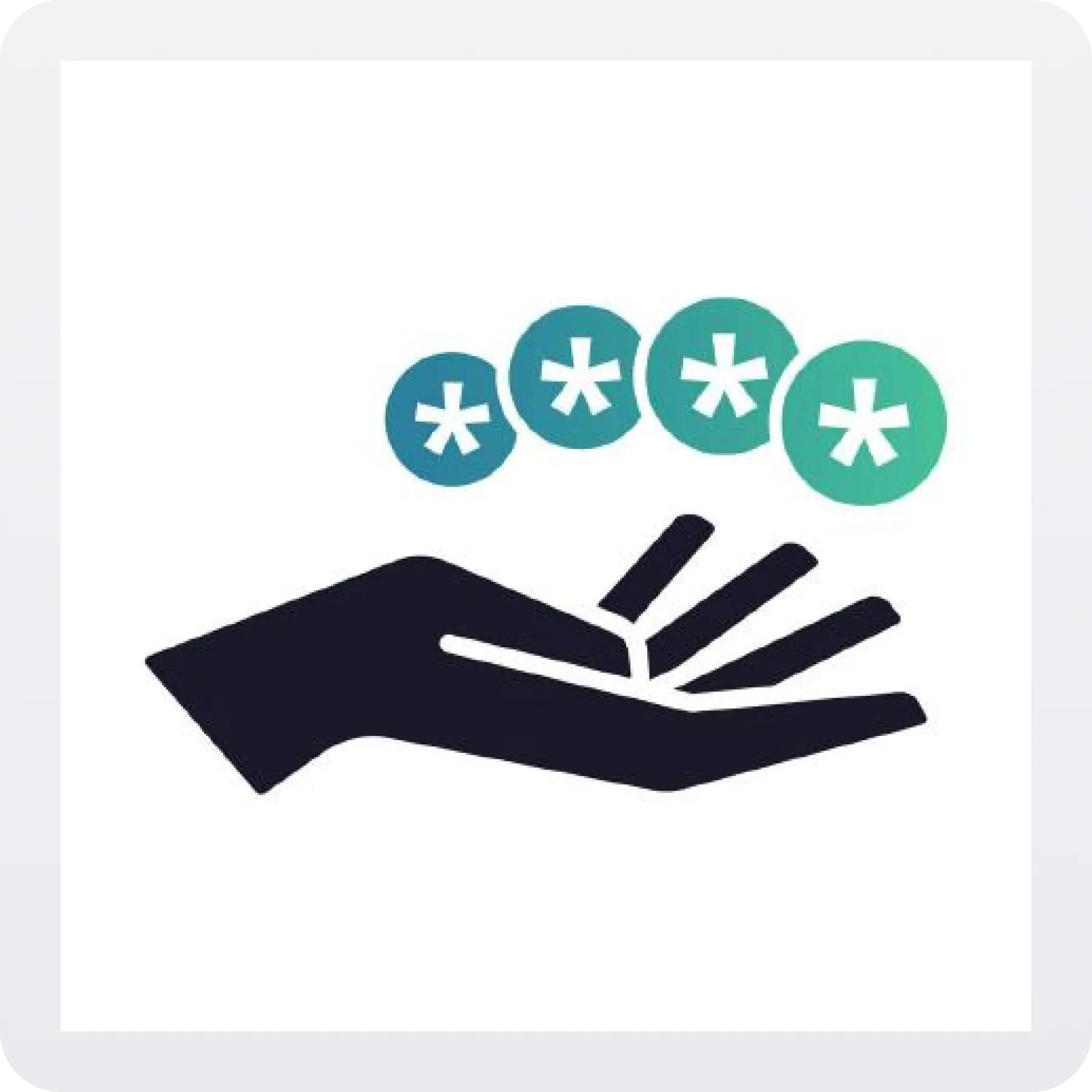
Sorcery is a minimalist, flexible authentication gem for Ruby on Rails that provides just the essential features needed to manage user authentication, sessions, and security. Like Authlogic, Sorcery is designed to give developers more control over the authentication process while keeping the system lightweight and simple. It is built for developers who want an easy-to-understand and highly customizable solution without the bloat of larger authentication gems like Devise.
Some of its key features are:
- Basic user authentication: sign up, sign in, and sign out with email and password. User passwords are securely hashed using a strong algorithm (typically bcrypt).
- Session management: persistent sessions (“remember me“ functionality), control over session expiration times and multiple session types for different use cases.
- Password reset via email.
- Although not built-in, Sorcery can be easily extended to manage roles and permissions by adding custom methods or integrating with gems like cancancan or pundit.
- Sorcery includes optional plugins for integrating third-party authentication via OmniAuth. This allows users to log in via Google, Facebook, GitHub, and other providers.
- Sorcery can be extended to support two-factor authentication (2FA) by integrating with third-party libraries or implementing your own custom 2FA solutions.
- You can extend or override the default authentication logic if you need special features like CAPTCHA verification, IP blocking, or custom user validation.
Sorcery is designed to be simple and straightforward. It doesn't include extra features you don’t need, which makes it ideal for applications that require a lean authentication solution.
Sorcery is a solid choice for developers who need a lightweight, flexible authentication solution for Ruby on Rails. It provides all the essential features for user authentication and session management, while being easily customizable to fit specific application needs. However, if you need advanced features like multi-factor authentication or social login out of the box, you may want to consider other options like Devise or OmniAuth.
Pundit: Minimal authorization through OO design and pure Ruby classes
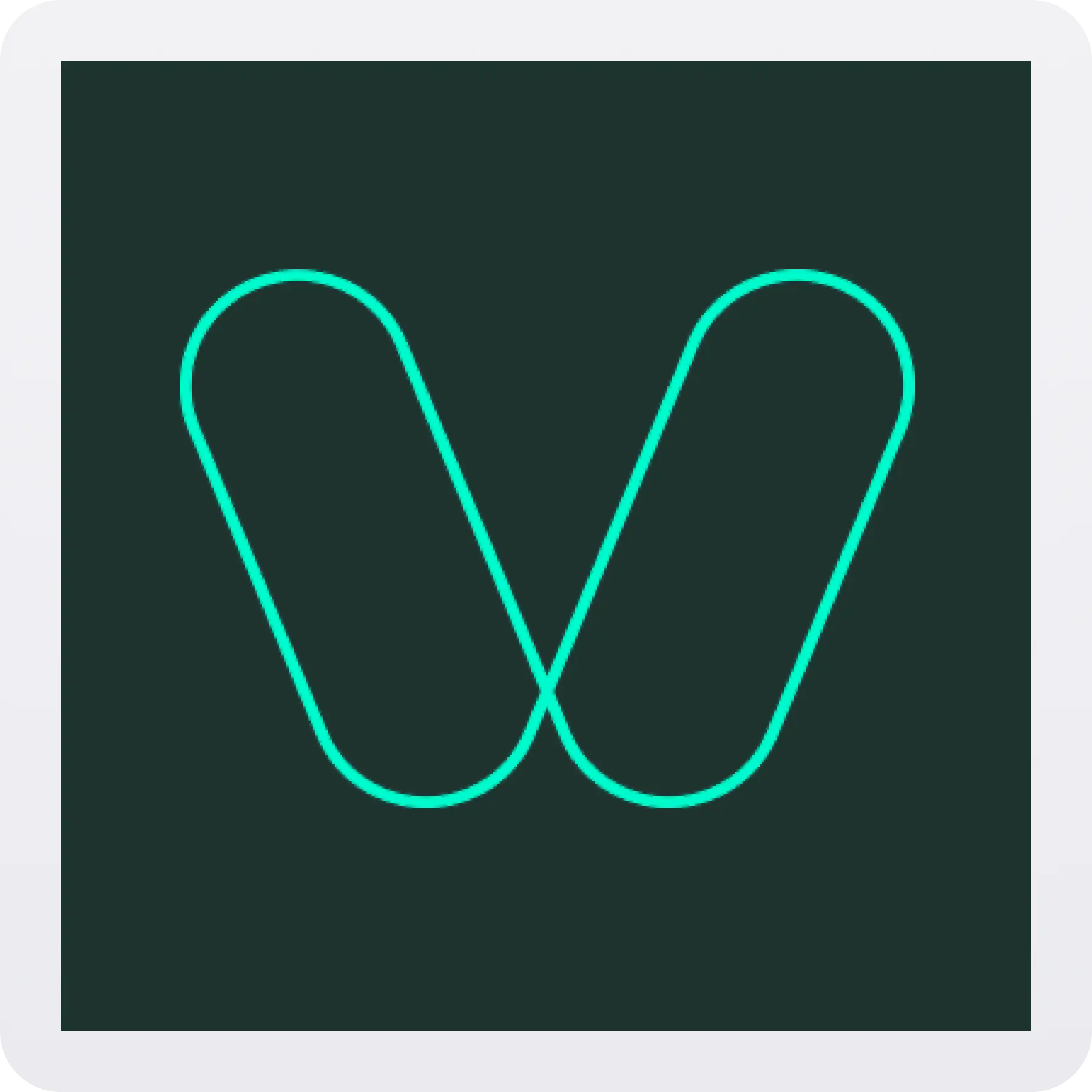
Pundit is a popular Ruby gem used for authorization in Rails applications. It helps you define policies that specify what a user is allowed to do with various resources (e.g., creating, updating, deleting records).
Pundit uses policies to encapsulate the rules for what actions a user can perform on a given resource. Policies are classes that define methods for each action (like create?, update?, destroy?) and return a boolean indicating whether the action is allowed. Policies can be customized based on the user's role, such as an admin, guest, or regular user. You can define different levels of access to resources based on user roles and other attributes.
This is an example that allows updating a post if the user is an admin, or if the post is unpublished:
Pundit encourages a clean separation of concerns by keeping authorization logic out of controllers and models. Instead, you place it in policy classes, making the code more modular, readable, and maintainable.
Pundit also allows you to define scopes that control which records a user can see. For example, you may want to limit the posts a user can see based on their role or ownership:
You can then use policy_scope
in your controller to get the appropriate records for the current user:
Pundit is an excellent choice for handling authorization in Ruby on Rails applications. It’s a lightweight, flexible solution that keeps your authorization logic separate from the rest of your application, making it easy to manage and extend. If you're looking for a simple way to define access control based on user roles, permissions, or attributes, Pundit is a great tool to add to your Rails project.
However, unlike gems like Devise, which bundle authentication and authorization together, Pundit only handles authorization, so you may need additional gems or code for authentication. Pundit also doesn’t come with predefined user roles or access control lists (ACLs), so you must implement your own role-based logic or integrate it with other libraries.
CanCanCan: Powerful and feature-rich authorization

CanCanCan is a popular and feature-rich gem for managing authorization in Ruby on Rails applications. It provides a simple, flexible way to define user permissions and manage access control based on roles or attributes. It’s particularly useful for applications with role-based access control (RBAC), where users have different levels of access based on their roles (e.g., admin, moderator, user). It integrates seamlessly with Rails and provides a clear and centralized way to manage authorization logic.
The gem is built on the idea of abilities, which determine what actions a user can perform on different resources (e.g., models or controllers) in your application. Abilities are stored in an Ability
class and are typically defined per model (e.g., Post
, User
, Article
).
You can set permissions on a per-action basis (e.g., read
, create
, update
, destroy
) and apply rules to specific resources or models. This allows for very granular control over what users can do.
For example, this class checks the current user's role and sets permissions accordingly. For instance, admins have full access (manage :all
), while regular users can only read and create posts.
Cancancan provides the ability to easily scope resources for users (e.g., showing only records that the current user is authorized to access). This can be particularly useful for limiting the visibility of resources based on user roles or ownership.
The gem uses a declarative interface for defining permissions, making it simple and intuitive to specify what users can do. You can easily grant or restrict access with methods like can
and cannot
within the Ability
class.
Cancancan integrates well with Rails controllers, views, and models. You can use helper methods like can?
, cannot?
, and authorize!
to enforce authorization checks and restrict access.
For example, the load_and_authorize_resource
method automatically loads the resource and checks if the user is authorized to access it.
The authorize!
method explicitly checks if the user has permission to perform a specific action.
In your views, you can use the can?
and cannot?
helper methods to show or hide UI elements based on the user's abilities:
You can define abilities dynamically based on user attributes or conditions. For example, a user may be allowed to edit an article if they are the author, but not if they are a guest.
Cancancan is a powerful, flexible, and easy-to-use gem for managing authorization in Ruby on Rails applications. It provides an intuitive way to define user permissions and roles, helping you control access to your resources. It can easily be integrated with other gems and libraries, such as Devise (for authentication), Pundit, and others, allowing for more complex use cases if needed.
Action Policy: Define policies on models or controllers
Action Policy is a Ruby gem designed to provide a simple, powerful, and flexible way to manage authorization in Rails applications. It allows you to define and enforce policies on your models or controllers, helping you manage user permissions in a clean and maintainable manner.
Unlike other authorization gems like CanCanCan or Pundit, Action Policy takes a more modern and object-oriented approach to authorization. It provides a clear and consistent way to manage user abilities and roles, and integrates well with Rails applications, offering rich features that make it easier to enforce permissions based on user roles, attributes, and other conditions.
Action Policy uses a straightforward and intuitive DSL (domain-specific language) for defining authorization rules, which makes it easy to implement and read. Policies are defined for specific resources or models, and you can easily configure actions like show
, create
, update
, and destroy
.
Policies are defined as Ruby classes. For example, if you have a Post
model and want to define authorization rules for it, you can create a policy like this:
In the example above, we define several actions (show
, create
, update
, destroy
) and set the rules for each action based on the current user and the resource (in this case, the Post
model).
You can configure the policy in your application’s controller by using the authorize
helper method to check whether a user is authorized to perform a particular action:
The authorize method checks whether the user has permission to perform the action (e.g., show
, create
, update
, destroy
) on the resource (@post
).
If you have more complex authorization needs, you can define multiple policies for the same resource, or even multiple policies that work together. For example, you could define policies for a resource based on different user roles.
Action Policy supports various advanced features like dynamic permissions, scope-based rules, and context-specific authorization, which allows you to customize your authorization logic to meet your application's unique needs.
Action Policy is an excellent gem for developers who need a flexible, modern, and maintainable solution for authorization in Ruby on Rails applications. Its clear syntax, support for role-based and resource-based permissions, and integration with Rails make it a great choice for both small and large applications. However, be aware of the learning curve that may come with complex permissions and contextual authorization rules.
Rolify: Basic RBAC for Rails apps

Rolify is a Ruby gem designed to manage roles and permissions within a Ruby on Rails application. It provides a simple way to assign roles to users and control access to resources based on those roles. Rolify integrates seamlessly with Rails and is often used in conjunction with other authentication gems, such as Devise or OmniAuth, to implement role-based access control (RBAC).
Users can have many roles, and roles can be assigned to many users. In addition to global roles, Rolify supports scoped roles, where roles can be defined for specific resources (e.g., a user may have a different role for a Project
than they do for a Blog
).
For example, to assign a :admin
role to a user:
To assign the :admin
role for a specific project:
Rolify provides helper methods to check if a user has a specific role, allowing you to easily query a user's roles in your application. You can use these methods to show or hide content based on the user's roles.
To check if a user has a specific role:
To find users with a role for a specific resource (e.g., a project), you can use:
On the downside, Rolify doesn't provide a built-in interface for managing roles and lacks built-in advanced features like hierarchical roles or granular permission management (which other gems like CanCanCan offer), and the project doesn't look actively maintained for some time.
Which one should I use?
The answer is—you guessed it—“it depends”. Each gem serves different needs, so the best choice depends on your project's complexity and requirements. The most feature-rich gem is not always the right choice if you need something up and running asap.
For authentication:
- If you're looking for a comprehensive, widely-used solution with a rich set of features out of the box, Devise is the go-to choice, offering easy configuration and robust security options.
- If you're interested in integrating third-party logins, OmniAuth is the perfect fit, providing seamless social login integrations.
- For a simpler, lightweight authentication system, Clearance or Authlogic are excellent choices, offering straightforward setups with enough customization options for small to medium-sized applications.
- If you need a more customizable solution with advanced features like multi-factor authentication and session management, Rodauth shines as a flexible, high-performance choice.
- For simpler or legacy projects, Sorcery remains a reliable option despite being unmaintained, but it’s best suited for small-scale applications. We would recommend, however, to avoid using unmaintained gems for security reasons.
On the authorization side:
- If you're building a permissions-based system with roles, CanCanCan provides an easy-to-understand DSL for defining user abilities, making it great for most applications.
- For fine-grained control and policy-based authorization, Pundit is a robust, object-oriented solution, offering a more explicit and flexible approach to handling user permissions.
- Rolify excels when you need to manage complex role structures with dynamic associations, making it ideal for applications requiring a flexible role management system.
Many applications use Devise for authentication and pair it with either Pundit or CanCanCan for authorization. Devise takes care of user authentication, while Pundit or CanCanCan manage user permissions and roles.
Another option is to use a third-party provider that will do most of the heavy lifting for you while keeping you and your users safe. WorkOS has a Ruby SDK and can cover all your authentication and authorization needs. Be it SSO, social logins, enterprise logins, RBAC, or fine-grained authorization, we have your back. And what’s more, you get up to 1,000,000 monthly active users for free.
Bonus gems
Here are some additional gems that we think you might find useful while securing your app:
- JWT is a Ruby implementation of the JWT spec and is used for encoding and decoding JSON Web Tokens (JWT). It supports both HMAC and RSA algorithms for signing tokens. It can be used to encode and decode JWTs, add custom claims to the token payload, set expiration times, and more.
- Secure Headers is a Ruby gem that automatically sets HTTP security headers to protect against a range of attacks. It helps prevent vulnerabilities like Cross-Site Scripting (XSS), Clickjacking, and other common exploits.
- Brakeman is a static analysis tool for Rails applications that scans your code for security vulnerabilities. It detects issues like SQL injection, Cross-Site Scripting (XSS), and mass assignment vulnerabilities.
- Rack::Attack provides a middleware for rate limiting and blocking abusive requests to your web application. It helps protect your app from malicious activities like brute-force attacks, DDoS, and other forms of abuse by allowing you to define custom throttling rules and blocking strategies based on request data such as IP addresses, paths, or headers.
- Figaro
- is a Ruby gem that simplifies the management of application configuration settings, especially sensitive ones like API keys and database credentials. It securely stores environment variables in a
config/application.yml
file and loads them into your app, making it easy to manage configuration across different environments without hardcoding sensitive data into your codebase. - Bullet helps you optimize your Rails application's database queries by identifying N+1 queries and unused eager loading. It provides helpful notifications during development to alert you about inefficient queries, allowing you to optimize your code for better performance and reduce unnecessary database load.